用python代码写文件in.txt (在作业附件里面)读取信息,文件里的单词中间由空格隔开,统计每个单词出现的次数,从大到小排序后输出到文件out.txt
时间: 2024-02-03 20:15:57 浏览: 82
可以使用Python中的字典来实现单词计数,然后使用sorted函数按照字典值从大到小排序。具体代码如下:
```python
# 读取文件内容
with open('in.txt', 'r') as f:
content = f.read()
# 统计单词出现次数
word_count = {}
for word in content.split():
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 按照单词出现次数从大到小排序
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
# 输出结果到文件
with open('out.txt', 'w') as f:
for word, count in sorted_word_count:
f.write(word + ' ' + str(count) + '\n')
```
这段代码会将处理后的结果输出到out.txt文件中。
相关问题
从文件in.txt(在作业附件里面)读取信息,文件里的单词中间由空格隔开,统计每个单词出现的次数,从大到小排序后输出到文件out.txt 【输入形式】 文件里存储了多个单词,有重复,空格隔开 【输出形式】 从大到小输出单词及其出现的次数,次数一样的单词按字符串比较从小到大排序 【输入样例】in.txt文件里面的数据如下所示: hello world python hello word file number file file python
以下是实现该功能的Python代码:
```python
# 读取文件内容
with open('in.txt', 'r') as f:
content = f.read()
# 将内容转换为单词列表
words = content.split()
# 统计每个单词出现的次数
word_count = {}
for word in words:
if word not in word_count:
word_count[word] = 1
else:
word_count[word] += 1
# 将单词及其出现次数转换为元组列表,并按次数从大到小、字符串从小到大排序
sorted_word_count = sorted(word_count.items(), key=lambda x: (-x[1], x[0]))
# 将排序后的结果输出到文件out.txt
with open('out.txt', 'w') as f:
for word, count in sorted_word_count:
f.write(f'{word} {count}\n')
```
这段代码首先读取文件内容,将其转换为单词列表,然后统计每个单词出现的次数,并将单词及其出现次数转换为元组列表。接着,使用`sorted()`函数按次数从大到小、字符串从小到大排序。最后,将排序后的结果输出到文件out.txt中。
从文件in.txt读取信息,文件里的单词中间由空格隔开,统计每个单词出现的次数,从大到小排序后输出到文件out.txt
好的,这个问题可以用 Python 来解决。你可以使用以下代码实现:
```python
# 读取文件内容
with open('in.txt', 'r') as f:
content = f.read()
# 将文件内容分割成单词列表
words_list = content.split()
# 统计每个单词出现的次数
word_count = {}
for word in words_list:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 按照单词出现次数从大到小排序
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
# 将排序后的结果输出到文件
with open('out.txt', 'w') as f:
for word, count in sorted_word_count:
f.write(f'{word} {count}\n')
```
这段代码会将 `in.txt` 文件中的单词读取出来,统计每个单词出现的次数,然后按照出现次数从大到小排序,最后将结果输出到 `out.txt` 文件中。
阅读全文
相关推荐
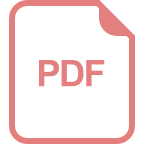
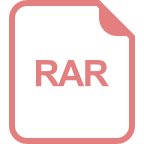
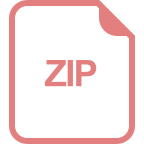
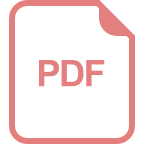
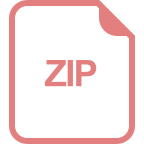
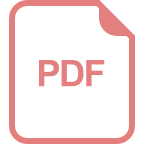
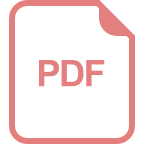
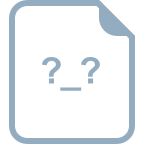
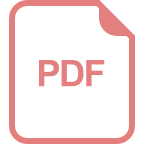
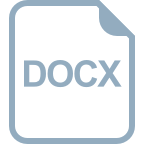
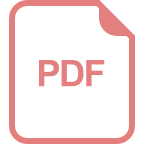