给51单片机连接esp8266WiFi模块和GPS模块,我让单片机通过WiFi模块将其接受到的GPS模块数据发送给电脑端用Python搭建的TCP通信,代码如何实现
时间: 2024-12-04 09:23:39 浏览: 12
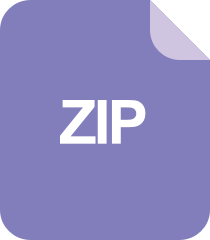
51驱动esp8266WiFi模块.zip_51驱动esp8266WiFi模块_WIFI串口_considervwc_ropeh
为51单片机连接ESP8266 WiFi模块和GPS模块,并通过TCP通信将GPS数据发送至Python服务器,你需要准备以下几个步骤:
**硬件连接**:
1. 将51单片机连接到ESP8266,一般通过UART接口(TX/RX)。
2. GPS模块的RX/TX分别连接到ESP8266的RX/TX接口。
3. ESP8266作为WiFi模块,连接到51单片机的SPI接口或专用的WiFi控制接口。
**软件部分**:
1. **51单片机部分**:
- 使用C语言编写51单片机代码,使用例如`u8g2`库(用于显示GPS数据)和`SoftwareSerial`库(用于连接GPS和ESP8266)。
```c
#include <SoftwareSerial.h>
SoftwareSerial gpsSerial(RX_PIN_GPS, TX_PIN_GPS);
#include "u8g2.h" // For displaying GPS on OLED or LCD
void setup() {
// Configure UART with ESP8266
// ...
// Initialize OLED/LCD display
u8g2.begin(U8G2_SSD1306_128X64_NONAME_F, &Serial1);
}
void loop() {
if (gpsSerial.available()) {
// Read GPS data and parse it
// Display GPS data on OLED/LCD
// ...
// When ready, send data over WiFi to Python server
sendDataToPython(gpsData);
}
}
// Function to send GPS data through TCP socket
void sendDataToPython(String gpsData) {
// Assuming you have a WiFi client connected to your network
WiFiClient client;
if (client.connect("your_server", YOUR_SERVER_PORT)) {
client.print("GPS Data: ");
client.println(gpsData);
client.stop(); // Close connection
}
}
```
2. **Python服务器部分**:
- 使用Python的`socketserver`库创建一个TCP服务器来监听来自ESP8266的数据。
```python
import socketserver
import sys
class SimpleTCPHandler(socketserver.BaseRequestHandler):
def handle(self):
data = self.request.recv(1024).strip()
print(f"Received from ESP: {data}")
# Save data or do further processing here
if __name__ == "__main__":
host, port = "localhost", YOUR_SERVER_PORT
server = socketserver.TCPServer((host, port), SimpleTCPHandler)
server.serve_forever()
```
**相关问题--:**
1. 如何确保51单片机和ESP8266的稳定通信?
2. 如何配置ESP8266连接到WiFi网络?
3. 若GPS数据发送失败,单片机如何处理这种情况?
阅读全文
相关推荐
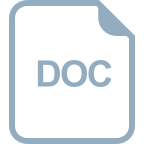
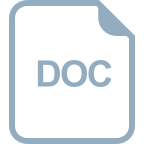
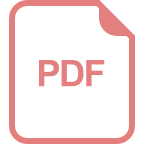
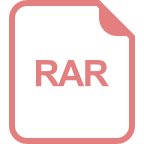
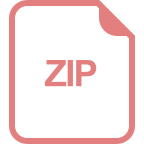
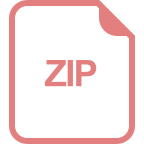
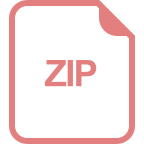
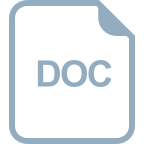
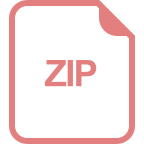
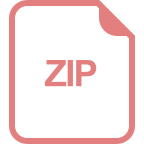
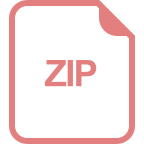
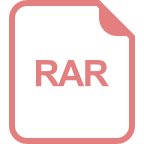
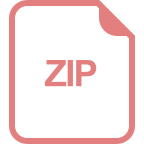
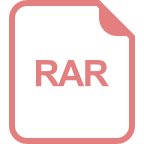
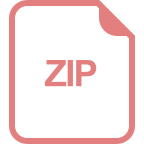
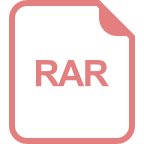