用vue做一个购物系统代码
时间: 2023-10-04 12:12:19 浏览: 63
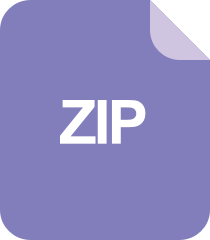
基于vue的商城购物系统
抱歉,由于购物系统代码比较复杂,超出了文本框的限制,无法在此处提供完整代码。不过,我可以提供一些实现购物系统的Vue代码结构和示例代码,供您参考:
1. 路由配置
在路由配置文件中,可以配置不同页面之间的路由,在路由跳转时,可以实现页面的无刷新跳转。
```javascript
import Vue from 'vue'
import Router from 'vue-router'
import Home from '@/components/Home'
import ProductList from '@/components/ProductList'
import ProductDetail from '@/components/ProductDetail'
import Cart from '@/components/Cart'
import Checkout from '@/components/Checkout'
import Login from '@/components/Login'
import Register from '@/components/Register'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'Home',
component: Home
},
{
path: '/products',
name: 'ProductList',
component: ProductList
},
{
path: '/products/:id',
name: 'ProductDetail',
component: ProductDetail
},
{
path: '/cart',
name: 'Cart',
component: Cart
},
{
path: '/checkout',
name: 'Checkout',
component: Checkout
},
{
path: '/login',
name: 'Login',
component: Login
},
{
path: '/register',
name: 'Register',
component: Register
}
]
})
```
2. 商品列表
在商品列表页面中,可以通过调用后端API获取商品信息,并且可以使用Vue组件来展示商品列表。
```javascript
<template>
<div class="product-list">
<h1>Product List</h1>
<div class="products">
<div v-for="product in products" :key="product.id">
<router-link :to="{name: 'ProductDetail', params: {id: product.id}}">
<img :src="product.image" :alt="product.name" />
<h2>{{ product.name }}</h2>
<p>{{ product.price }}</p>
</router-link>
</div>
</div>
</div>
</template>
<script>
import axios from 'axios'
export default {
name: 'ProductList',
data () {
return {
products: []
}
},
mounted () {
axios.get('/api/products')
.then(response => {
this.products = response.data
})
.catch(error => {
console.log(error)
})
}
}
</script>
```
3. 商品详情
在商品详情页面中,可以展示商品的详细信息,并且可以加入购物车。
```javascript
<template>
<div class="product-detail">
<img :src="product.image" :alt="product.name" />
<h2>{{ product.name }}</h2>
<p>{{ product.price }}</p>
<button @click="addToCart">Add to Cart</button>
</div>
</template>
<script>
import { mapActions } from 'vuex'
import axios from 'axios'
export default {
name: 'ProductDetail',
data () {
return {
product: {}
}
},
mounted () {
axios.get(`/api/products/${this.$route.params.id}`)
.then(response => {
this.product = response.data
})
.catch(error => {
console.log(error)
})
},
methods: {
...mapActions(['addToCart']),
addToCart () {
this.addToCart({id: this.product.id, name: this.product.name, price: this.product.price})
}
}
}
</script>
```
4. 购物车
在购物车页面中,可以展示用户已经选择的商品,并且可以进行结算操作。
```javascript
<template>
<div class="cart">
<h1>Shopping Cart</h1>
<div class="cart-items">
<div v-for="item in cartItems" :key="item.id">
<div>{{ item.name }}</div>
<div>{{ item.price }}</div>
<div>
<button @click="incrementItem(item.id)">+</button>
{{ item.quantity }}
<button @click="decrementItem(item.id)">-</button>
</div>
<div>{{ item.price * item.quantity }}</div>
<div><button @click="removeItem(item.id)">Remove</button></div>
</div>
</div>
<h2>Total: {{ total }}</h2>
<button @click="checkout">Checkout</button>
</div>
</template>
<script>
import { mapGetters, mapActions } from 'vuex'
export default {
name: 'Cart',
computed: {
...mapGetters(['cartItems', 'total'])
},
methods: {
...mapActions(['incrementItem', 'decrementItem', 'removeItem', 'checkout'])
}
}
</script>
```
以上是购物系统的一些Vue代码结构和示例代码,希望能够帮助您开发购物系统。如果您有其他问题或者需要更详细的代码指导,请告诉我,我会尽力为您提供帮助。
阅读全文
相关推荐
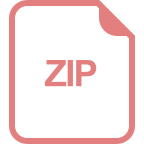

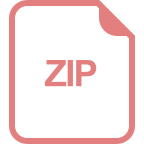
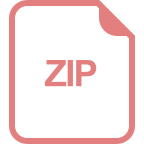
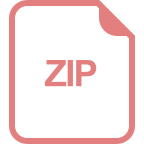
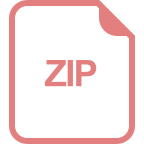
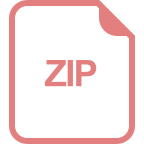
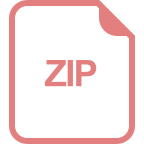
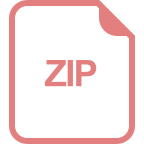
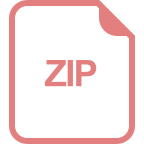
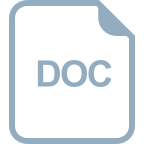
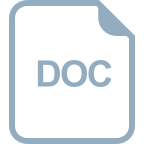
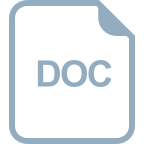
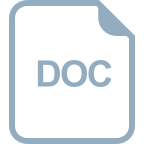
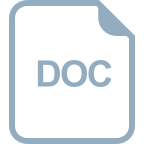
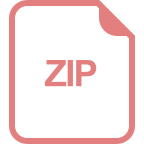