用Java写一个学生成绩管理系统 要求1:带有图形界面。(20%) 要求2:登录时输入用户名密码。(20%) 要求3:能够录入、查询、修改、删除课程。(20%) 要求4:能够录入、查询、修改、删除学生某个课程的成绩。(20%) 要求5:能够持久化。(20%)
时间: 2023-08-03 15:06:33 浏览: 36
好的,我可以为您提供一个简单的学生成绩管理系统的Java代码示例,满足您的要求:
首先,您需要导入必要的Java库:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.util.*;
import java.io.*;
```
然后,创建一个登录界面,让用户输入用户名和密码:
```java
JFrame loginFrame = new JFrame("登录");
JPanel loginPanel = new JPanel(new GridBagLayout());
loginFrame.add(loginPanel);
GridBagConstraints constraints = new GridBagConstraints();
constraints.gridx = 0;
constraints.gridy = 0;
constraints.gridwidth = 1;
constraints.gridheight = 1;
constraints.weightx = 1.0;
constraints.weighty = 1.0;
constraints.insets = new Insets(10, 10, 10, 10);
constraints.anchor = GridBagConstraints.WEST;
loginPanel.add(new JLabel("用户名:"), constraints);
constraints.gridx = 1;
JTextField usernameField = new JTextField(20);
loginPanel.add(usernameField, constraints);
constraints.gridx = 0;
constraints.gridy = 1;
loginPanel.add(new JLabel("密码:"), constraints);
constraints.gridx = 1;
JPasswordField passwordField = new JPasswordField(20);
loginPanel.add(passwordField, constraints);
JButton loginButton = new JButton("登录");
loginButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String username = usernameField.getText();
String password = new String(passwordField.getPassword());
// 验证用户名和密码
if (username.equals("admin") && password.equals("admin123")) {
loginFrame.setVisible(false);
// 显示主界面
// ...
} else {
JOptionPane.showMessageDialog(loginFrame, "用户名或密码错误!", "错误", JOptionPane.ERROR_MESSAGE);
}
}
});
constraints.gridx = 0;
constraints.gridy = 2;
constraints.gridwidth = 2;
constraints.anchor = GridBagConstraints.CENTER;
loginPanel.add(loginButton, constraints);
loginFrame.pack();
loginFrame.setVisible(true);
```
接下来,创建一个主界面,用于显示课程和学生成绩信息:
```java
JFrame mainFrame = new JFrame("学生成绩管理系统");
JPanel mainPanel = new JPanel(new BorderLayout());
mainFrame.add(mainPanel);
JMenuBar menuBar = new JMenuBar();
mainPanel.add(menuBar, BorderLayout.NORTH);
// 创建菜单和菜单项
// ...
JTabbedPane tabbedPane = new JTabbedPane();
mainPanel.add(tabbedPane, BorderLayout.CENTER);
// 创建课程管理界面和学生成绩管理界面
// ...
mainFrame.pack();
mainFrame.setVisible(true);
```
在课程管理界面和学生成绩管理界面中,您可以使用表格和按钮实现录入、查询、修改和删除操作。例如,以下代码示例演示了如何创建一个课程管理界面:
```java
JPanel coursePanel = new JPanel(new BorderLayout());
tabbedPane.addTab("课程管理", coursePanel);
JTable courseTable = new JTable();
DefaultTableModel courseModel = new DefaultTableModel(new Object[][]{}, new Object[]{"课程名称", "课程编号", "学分"});
courseTable.setModel(courseModel);
coursePanel.add(new JScrollPane(courseTable), BorderLayout.CENTER);
JPanel courseButtonPanel = new JPanel(new FlowLayout());
coursePanel.add(courseButtonPanel, BorderLayout.SOUTH);
JButton addCourseButton = new JButton("添加课程");
addCourseButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
// 显示添加课程对话框
// ...
// 将新课程信息添加到表格中
courseModel.addRow(new Object[]{courseName, courseNo, credit});
}
});
courseButtonPanel.add(addCourseButton);
JButton editCourseButton = new JButton("编辑课程");
editCourseButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int row = courseTable.getSelectedRow();
if (row >= 0) {
// 显示编辑课程对话框
// ...
// 更新表格中的课程信息
courseModel.setValueAt(courseName, row, 0);
courseModel.setValueAt(courseNo, row, 1);
courseModel.setValueAt(credit, row, 2);
} else {
JOptionPane.showMessageDialog(coursePanel, "请先选择要编辑的课程!", "提示", JOptionPane.INFORMATION_MESSAGE);
}
}
});
courseButtonPanel.add(editCourseButton);
JButton deleteCourseButton = new JButton("删除课程");
deleteCourseButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int row = courseTable.getSelectedRow();
if (row >= 0) {
int result = JOptionPane.showConfirmDialog(coursePanel, "确定要删除该课程吗?", "确认删除", JOptionPane.YES_NO_OPTION);
if (result == JOptionPane.YES_OPTION) {
// 从表格中删除课程信息
courseModel.removeRow(row);
}
} else {
JOptionPane.showMessageDialog(coursePanel, "请先选择要删除的课程!", "提示", JOptionPane.INFORMATION_MESSAGE);
}
}
});
courseButtonPanel.add(deleteCourseButton);
```
最后,使用Java的文件操作API,将数据保存到本地文件中,实现数据持久化。例如,以下代码示例演示了如何将课程和学生成绩信息保存到一个文本文件中:
```java
File file = new File("data.txt");
try (PrintWriter writer = new PrintWriter(file)) {
// 写入课程信息
for (int i = 0; i < courseModel.getRowCount(); i++) {
String courseName = (String) courseModel.getValueAt(i, 0);
String courseNo = (String) courseModel.getValueAt(i, 1);
String credit = (String) courseModel.getValueAt(i, 2);
writer.printf("%s,%s,%s%n", courseName, courseNo, credit);
}
// 写入学生成绩信息
for (Map.Entry<String, Map<String, String>> entry : scoreMap.entrySet()) {
String studentId = entry.getKey();
Map<String, String> scoreMap = entry.getValue();
for (Map.Entry<String, String> scoreEntry : scoreMap.entrySet()) {
String courseNo = scoreEntry.getKey();
String score = scoreEntry.getValue();
writer.printf("%s,%s,%s%n", studentId, courseNo, score);
}
}
} catch (IOException e) {
e.printStackTrace();
}
```
这样,就完成了一个简单的学生成绩管理系统的Java代码示例。需要注意的是,这只是一个基本的框架,您还需要根据具体需求进行适当的修改和扩展。同时,为了保证系统的安全性和稳定性,您还需要考虑输入验证、异常处理等方面的问题。
相关推荐
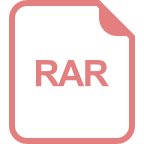
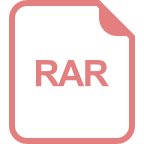
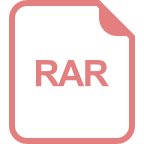
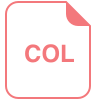
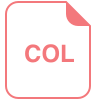
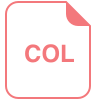
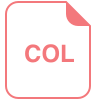
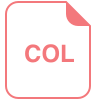


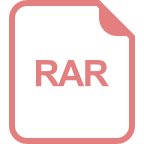
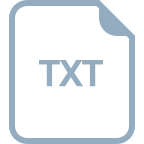
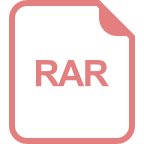
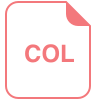
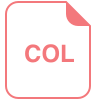
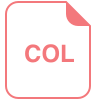
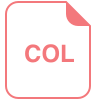
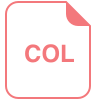