无需引入 pixelmatch 库,用vue实现图片找不同小游戏整套代码
时间: 2024-01-27 21:05:26 浏览: 23
好的,以下是一个使用Vue实现图片找不同小游戏的示例代码:
```html
<template>
<div class="game-container">
<div class="image-container">
<img :src="images[0]" class="image">
<img :src="images[1]" class="image difference" @click="checkDifference">
</div>
<div class="feedback-container">
<div v-if="differenceFound" class="feedback correct">Correct!</div>
<div v-if="!differenceFound && differenceChecked" class="feedback incorrect">Incorrect, please try again.</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
images: [
"https://example.com/image1.png",
"https://example.com/image2.png"
],
differenceFound: false,
differenceChecked: false
};
},
methods: {
checkDifference() {
// Create two image objects
const img1 = new Image();
const img2 = new Image();
img1.src = this.images[0];
img2.src = this.images[1];
// Wait for both images to load
Promise.all([img1, img2].map(img => new Promise(resolve => img.onload = resolve)))
.then(() => {
// Create a canvas to draw the images onto
const canvas = document.createElement("canvas");
canvas.width = img1.width;
canvas.height = img1.height;
// Draw the images onto the canvas
const ctx = canvas.getContext("2d");
ctx.drawImage(img1, 0, 0);
ctx.drawImage(img2, 0, 0);
// Get the pixel data for both images
const data1 = ctx.getImageData(0, 0, img1.width, img1.height).data;
const data2 = ctx.getImageData(0, 0, img2.width, img2.height).data;
// Check if there are any differences between the images
let differenceFound = false;
for (let i = 0; i < data1.length; i += 4) {
const r1 = data1[i];
const g1 = data1[i + 1];
const b1 = data1[i + 2];
const r2 = data2[i];
const g2 = data2[i + 1];
const b2 = data2[i + 2];
if (r1 !== r2 || g1 !== g2 || b1 !== b2) {
differenceFound = true;
break;
}
}
// Update the state with the result
if (differenceFound) {
this.differenceChecked = true;
} else {
this.differenceFound = true;
}
});
}
}
};
</script>
<style>
.game-container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
}
.image-container {
position: relative;
}
.image {
max-width: 100%;
}
.difference {
position: absolute;
top: 0;
left: 0;
cursor: pointer;
}
.feedback-container {
margin-top: 20px;
}
.feedback {
font-size: 18px;
padding: 10px;
border-radius: 5px;
}
.correct {
background-color: green;
color: white;
}
.incorrect {
background-color: red;
color: white;
}
</style>
```
这个示例代码中,我们使用了两张图片来实现找不同游戏。其中,一张图片是原图,另一张图片是在原图上添加了一个差异的图片。通过点击添加了差异的图片来检查是否找到了差异。如果找到了差异,则显示“Correct!”的反馈,否则显示“Incorrect, please try again.”的反馈。
在checkDifference方法中,我们使用了HTML5 Canvas来比较两张图片的像素,以检查它们之间是否存在差异。如果没有差异,则将differenceFound设置为true,否则将differenceChecked设置为true,以便在用户再次尝试时显示错误的反馈。
需要注意的是,这个示例代码中图片的URL是假的,需要替换成你自己的图片URL。
相关推荐
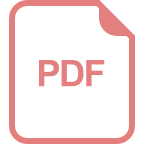














