5、(基础题)参照上一题的程序,掌握用类成员函数重载运算符的方法 要求:在下列程序的适当位置添加代码,使用成员函数方法实现下列运算符重载: (1) 复数 + 实数(double类型) 运算; (2) 复数 * 复数 运算。 程序代码: #include <iostream> using namespace std; class Complex { private: double real,image; //分别为实部、虚部 public: Complex(double rel=0, double img=0) { real=rel; image=img; } void display() //输出(a+b*i) 、(a-b*i) 、(a)等形式 { cout<<" ("<<real; if (image>0) cout<<"+"<<image<<"*i) "; //虚部为正 else if (image<0) cout<<image<<"*i) "; //虚部为负 else cout<<") "; //虚部为0,即为实数 } Complex operator +(Complex & c); //用成员函数重载 }; //成员函数的类外定义 Complex Complex::operator +(Complex & c) { Complex temp; temp.real=real+c.real; temp.image=image+c.image; return temp; } int main() { Complex c1(1,2), c2(3,-4),c3,c4; c3=c1+123.456; c4=c1*c2; //输出加的结果 c1.display(); cout<<"+"; cout<<123.456; cout<<"="; c3.display(); cout<<endl; //输出*的结果 c1.display(); cout<<"*"; c2.display(); cout<<"="; c4.display (); cout<<endl; return 0; }
时间: 2024-01-27 13:05:01 浏览: 67
在类定义中添加以下成员函数:
```
Complex operator +(double num);
Complex operator *(Complex & c);
```
在类外定义中实现:
```
Complex Complex::operator +(double num) {
Complex temp;
temp.real = real + num;
temp.image = image;
return temp;
}
Complex Complex::operator *(Complex & c) {
Complex temp;
temp.real = real * c.real - image * c.image;
temp.image = real * c.image + image * c.real;
return temp;
}
```
在 `main` 函数中调用:
```
c3 = c1 + 123.456;
c4 = c1 * c2;
```
其中,`c1 + 123.456` 调用了 `Complex::operator +(double num)`,`c1 * c2` 调用了 `Complex::operator *(Complex & c)`。
相关问题
定义一个复数类complex,重载运算符“+”,使之能用于复数的加法运算。将运算符函数重载为非成员、非友元的普通函数。编写程序,求两个复数之和,并传调试结果。
题目描述:定义一个复数类 `complex`,重载运算符“+”,使之能用于复数的加法运算。将运算符函数重载为非成员、非友元的普通函数。编写程序,求两个复数之和,并传调试结果。
解析:该题要求定义一个复数类 `complex` 并重载加法运算符 “+”,使之能够作为复数的加法运算。并将运算符函数重载为非成员、非友元的普通函数。最后,编写程序,并求出两个复数之和,将调试结果进行传递。
阅读全文
相关推荐
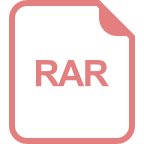
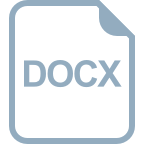
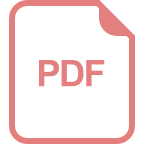
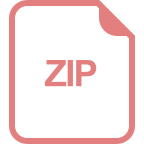
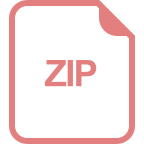
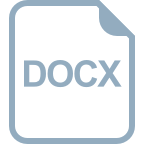
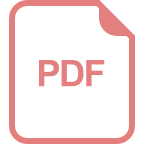
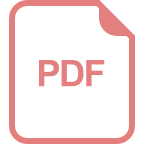
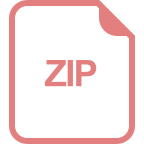
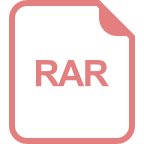
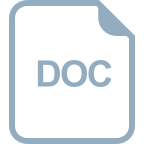
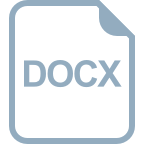
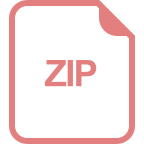