请在LDA模型一致性计算中使用'u_mass'来获得最快的速度,并只需要'u_mass'的模型、语料库和一致性参数。,使用python语言
时间: 2024-05-16 16:15:56 浏览: 213
好的,以下是使用Python计算LDA模型一致性的代码示例:
```python
from gensim.models import LdaModel
from gensim.corpora import Dictionary
from gensim.models.coherencemodel import CoherenceModel
# 加载语料库
corpus = YOUR_CORPUS
# 加载字典
dictionary = Dictionary.load(YOUR_DICTIONARY)
# 加载LDA模型
lda_model = LdaModel.load(YOUR_LDA_MODEL)
# 计算一致性
coherence_model_lda = CoherenceModel(model=lda_model, corpus=corpus, dictionary=dictionary, coherence='u_mass')
coherence_score = coherence_model_lda.get_coherence()
print("LDA模型一致性得分:", coherence_score)
```
其中,`YOUR_CORPUS`是你的语料库,`YOUR_DICTIONARY`是你的字典,`YOUR_LDA_MODEL`是你的LDA模型文件路径。在计算一致性时,我们使用了`'u_mass'`参数来获得最快的速度。最后输出的`coherence_score`就是LDA模型的一致性得分。
相关问题
给你一个已经分好词和去完停用词的f1.txt文件,请在LDA模型一致性计算中使用'u_mass'来获得最快的速度,并只需要'u_mass'的模型、语料库和一致性参数,并把图形运行出来保存起来,使用python语言
好的,我们可以使用gensim库来进行LDA模型的训练和一致性计算。首先,我们需要准备好语料库和停用词表。
假设我们的f1.txt文件已经分好词和去完停用词,每个词之间以空格隔开,那么我们可以按照以下方式读取文件并生成语料库:
```python
from gensim import corpora
# 读取文件,每行为一个文档,每个词以空格隔开
with open('f1.txt', 'r', encoding='utf-8') as f:
docs = [line.strip().split() for line in f]
# 生成词典
dictionary = corpora.Dictionary(docs)
# 生成文档向量
corpus = [dictionary.doc2bow(doc) for doc in docs]
```
接下来,我们可以使用u_mass参数训练LDA模型,并计算一致性得分:
```python
from gensim.models import CoherenceModel, LdaModel
# 训练LDA模型,设置主题数为10
lda_model = LdaModel(corpus=corpus, id2word=dictionary, num_topics=10, passes=10)
# 计算一致性得分
coherence_model_lda = CoherenceModel(model=lda_model, corpus=corpus, dictionary=dictionary, coherence='u_mass')
coherence_lda = coherence_model_lda.get_coherence()
print('一致性得分:', coherence_lda)
```
最后,我们可以使用matplotlib库来绘制一致性得分随着主题数变化的趋势图:
```python
import matplotlib.pyplot as plt
# 绘制一致性得分趋势图
topics = range(2, 20, 2)
coherence_score = []
for k in topics:
lda_model = LdaModel(corpus=corpus, id2word=dictionary, num_topics=k, passes=10)
coherence_model_lda = CoherenceModel(model=lda_model, corpus=corpus, dictionary=dictionary, coherence='u_mass')
coherence_lda = coherence_model_lda.get_coherence()
coherence_score.append(coherence_lda)
plt.plot(topics, coherence_score)
plt.xlabel("主题数")
plt.ylabel("一致性得分")
plt.show()
```
这样就可以得到一致性得分随着主题数变化的趋势图了。
lda模型语料选择代码
LDA模型语料选择的代码可以参考以下步骤:
1. 准备语料库,可以使用Python的gensim库进行处理,将文本转化为向量形式。
```python
from gensim import corpora
# 读取文本文件
docs = []
with open('corpus.txt', mode='r', encoding='utf-8') as f:
for line in f:
docs.append(line.strip())
# 构建词典
dictionary = corpora.Dictionary([doc.split() for doc in docs])
# 将文本转化为向量形式
corpus = [dictionary.doc2bow(doc.split()) for doc in docs]
```
2. 进行LDA模型训练,可以使用Python的gensim库进行训练。
```python
from gensim.models.ldamodel import LdaModel
# 训练LDA模型
lda_model = LdaModel(corpus=corpus, id2word=dictionary, num_topics=10, passes=10)
```
在训练过程中,可以根据实际需要设置num_topics和passes参数,分别表示主题数和迭代次数。
3. 查看LDA模型结果,可以使用Python的gensim库进行查看。
```python
# 查看主题-词分布
for topic_id in range(lda_model.num_topics):
print('Topic', topic_id)
print(lda_model.show_topic(topic_id))
# 查看文档-主题分布
for i in range(len(corpus)):
print('Document', i)
print(lda_model[corpus[i]])
```
通过以上代码,可以得到LDA模型训练的结果,进而选择合适的语料库进行模型训练和应用。
阅读全文
相关推荐
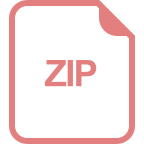
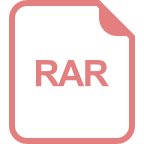
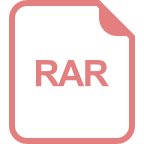
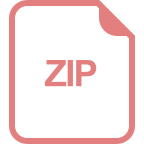
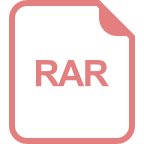
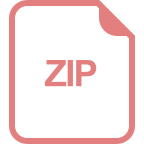
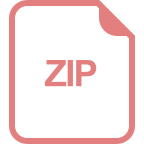
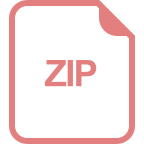
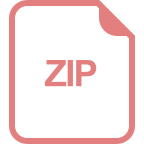
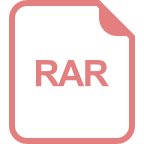
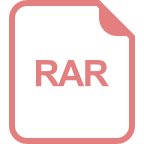
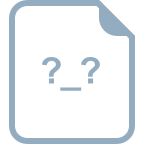