pyqt5添加背景图片
时间: 2024-01-25 15:11:54 浏览: 42
在PyQt5中添加背景图片有两种方法:
方法一:使用setStyleSheet()方法设置背景图片
```python
self.button.setStyleSheet("QPushButton{background-image: url(img/1.png)}")
```
方法二:使用QPalette类设置背景图片
```python
from PyQt5.QtGui import QPalette, QPixmap
# 创建一个QPalette对象
palette = QPalette()
# 加载背景图片
background_image = QPixmap("img/1.png")
# 设置背景图片为按钮的背景
palette.setBrush(QPalette.Background, background_image)
# 将QPalette对象应用到按钮上
self.button.setPalette(palette)
```
这两种方法都可以实现在PyQt5中添加背景图片的效果。第一种方法使用setStyleSheet()方法直接设置背景图片,但无法设置边框。第二种方法使用QPalette类设置背景图片,并且可以设置边框。
相关问题
pyqt5 添加背景图片
可以使用QPixmap和QPalette两个类来实现在PyQt5中添加背景图片的效果。
下面是一个简单的示例代码:
```python
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QWidget, QVBoxLayout, QApplication
from PyQt5.QtGui import QPalette
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
#设置窗口大小
self.setGeometry(300, 300, 600, 400)
#设置窗口标题
self.setWindowTitle('Background image')
#创建一个垂直布局
vbox = QVBoxLayout(self)
#创建一个标签,并设置背景图片
label = QLabel(self)
pixmap = QPixmap('your_image_path')
label.setPixmap(pixmap)
label.setScaledContents(True)
#将标签添加到布局中
vbox.addWidget(label)
#设置背景
pal = QPalette()
pal.setBrush(self.backgroundRole(), QBrush(pixmap))
self.setPalette(pal)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
ex.show()
sys.exit(app.exec_())
```
在上面的代码中,我们首先创建了一个QPixmap对象,并将其设置为一个QLabel对象的背景图片。然后,我们设置QLabel的内容自适应窗口大小,以保证图片完全显示。
接着,我们创建了一个QPalette对象,并将其设置为窗口的背景,从而实现了在PyQt5中添加背景图片的效果。
python pyqt5 添加背景图片
可以使用QPixmap和QPalette类来添加背景图片,具体实现可以参考以下代码:
```python
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtGui import QPalette
class MyWidget(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 设置窗口大小和标题
self.setGeometry(300, 300, 300, 220)
self.setWindowTitle('添加背景图片')
# 加载背景图片
palette = QPalette()
pix = QPixmap('background.jpg')
palette.setBrush(QPalette.Background, pix)
self.setPalette(palette)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyWidget()
ex.show()
sys.exit(app.exec_())
```
其中,'background.jpg'是你要添加的背景图片的路径。
相关推荐
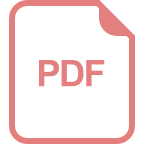












