设计密码校验函数:长度8-16之间,大写英文字符、小写英文字符、数字、特殊字符必须都有。将这些字符检验函数全部保存为一个字符检验模块(文件),在其它程序中导入这个字符检验模块,可以直接使用其内的所有字符校验函数。(程序代码截图)
时间: 2024-02-19 08:58:10 浏览: 124
好的,我可以为您设计一个密码校验函数,并将其与其他字符检验函数保存在一个模块中。以下是该模块的Python实现:
```python
import re
def check_length(password):
return 8 <= len(password) <= 16
def check_uppercase(password):
return bool(re.search(r'[A-Z]', password))
def check_lowercase(password):
return bool(re.search(r'[a-z]', password))
def check_digit(password):
return bool(re.search(r'\d', password))
def check_special(password):
return bool(re.search(r'[!@#$%^&*()_+-=\\\[\]{}|;\':",./<>?]', password))
def check_password(password):
return all([
check_length(password),
check_uppercase(password),
check_lowercase(password),
check_digit(password),
check_special(password)
])
```
该模块包含了以下函数:
- `check_length`:检查密码长度是否在8到16之间。
- `check_uppercase`:检查密码中是否包含大写英文字母。
- `check_lowercase`:检查密码中是否包含小写英文字母。
- `check_digit`:检查密码中是否包含数字。
- `check_special`:检查密码中是否包含特殊字符。
- `check_password`:综合检查密码是否符合要求,即密码长度在8到16之间,且包含大写英文字母、小写英文字母、数字、特殊字符。
在其他程序中,您可以通过导入该模块并调用其中的函数来进行字符校验,例如:
```python
import character_check
password = input('请输入密码:')
if character_check.check_password(password):
print('密码合法')
else:
print('密码不合法')
```
请注意,由于本模块使用了正则表达式进行校验,因此在使用前需要导入re模块。
阅读全文
相关推荐
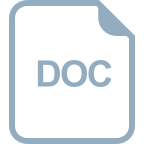
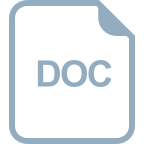
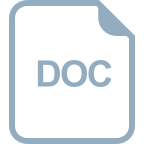




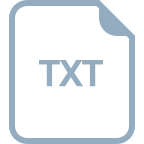
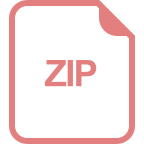
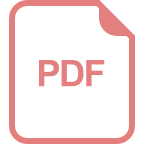








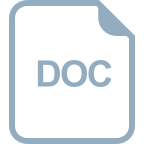