C++ thread函数
时间: 2023-08-25 22:07:07 浏览: 97
在C++中,std::thread类用于创建和管理线程。它有多个构造函数,其中一些常用的构造函数有以下几种:
1. thread():默认构造函数,创建一个空的线程对象。
2. thread(thread&& other):移动构造函数,用于将一个线程对象的所有权转移到另一个线程对象。
3. template<class Function, class... Args> explicit thread(Function&& f, Args&&... args):带有函数和参数的构造函数,用于创建一个新线程并执行指定的函数。
4. thread(const thread&)=delete:禁用的拷贝构造函数,确保线程对象不能被复制。
以下是一个使用std::thread创建线程的简单例子,其中调用thread对象的构造函数来执行指定的函数,并通过join()函数等待线程执行完成:
```cpp
#include <iostream>
#include <thread>
void myfunc() {
std::cout << "myfunc\n";
// do something ...
}
int main() {
std::thread t1(myfunc);
t1.join();
return 0;
}
```
如果想要使用std::thread创建多个线程,可以将thread对象存储在vector容器中,并使用循环来创建和加入线程:
```cpp
#include <iostream>
#include <thread>
#include <vector>
void foo(int n) {
std::cout << "foo() " << n << std::endl;
}
int main() {
std::vector<std::thread> threads;
for (int i = 0; i < 3; i++) {
threads.push_back(std::thread(foo, i));
}
for (int i = 0; i < 3; i++) {
threads[i].join();
}
std::cout << "main() exit.\n";
return 0;
}
```
在参数传递方面,可以使用传引用的方式将参数传递给线程函数。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [C++ thread用法总结(整理)](https://blog.csdn.net/fuhanghang/article/details/114818215)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [C++中的thread最全用法归纳](https://blog.csdn.net/zzh516451964zzh/article/details/128421574)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
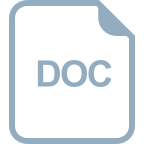
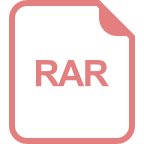
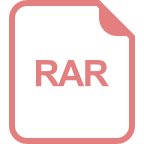
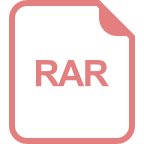
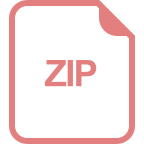
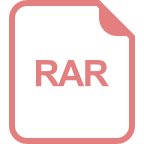
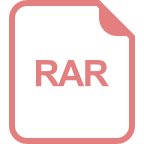
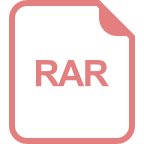
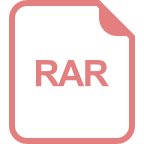
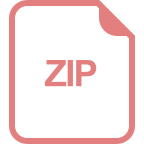
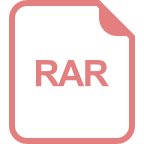
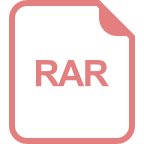
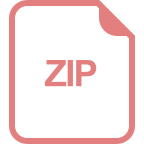
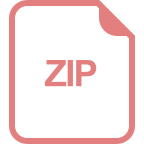
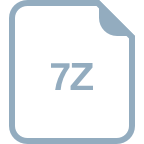
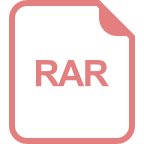
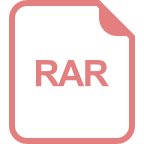