WPF BitmapSource 创建指定uri路径
时间: 2023-12-03 07:42:56 浏览: 46
你可以使用以下代码来创建指定 URI 路径的 `BitmapSource`:
```csharp
Uri uri = new Uri("your image uri here");
BitmapImage bitmap = new BitmapImage();
bitmap.BeginInit();
bitmap.UriSource = uri;
bitmap.CacheOption = BitmapCacheOption.OnLoad;
bitmap.EndInit();
BitmapSource bitmapSource = bitmap as BitmapSource;
```
在这个代码中,我们首先创建一个 `Uri` 对象,该对象指定了图像文件的路径。然后,我们使用 `BitmapImage` 类加载图像文件,并将 `Uri` 路径指定为 `UriSource` 属性的值。我们还将 `CacheOption` 属性设置为 `BitmapCacheOption.OnLoad`,以确保图像在加载时立即缓存。
最后,我们将 `BitmapImage` 对象转换为 `BitmapSource` 对象,以便在 WPF 应用程序中使用。
相关问题
wpf中如何用绝对路径创建一个uri对象
在 WPF 中,可以使用以下代码创建绝对路径的 Uri 对象:
```csharp
Uri uri = new Uri("pack://application:,,,/YourFolder/YourFile.xaml");
```
其中,`YourFolder` 是你的文件所在的文件夹名称,`YourFile.xaml` 是你的文件名称。请注意,`pack://application:,,,/` 表示应用程序根目录。如果你的文件不在根目录下,你需要相应地修改路径。
另外,如果你需要加载一个外部文件,例如本地磁盘上的文件,你可以使用以下代码:
```csharp
Uri uri = new Uri(@"file:///C:/YourFolder/YourFile.xaml");
```
其中,`C:/YourFolder/YourFile.xaml` 是你的文件路径。注意,路径中的斜杠必须使用正斜杠 `/`,否则需要使用转义字符 `\\`。
WPF 如何调用指定路径下的DLL
在 WPF 中调用指定路径下的 DLL,可以使用 `Assembly.LoadFrom` 方法加载 DLL。具体步骤如下:
1. 引用 `System.Reflection` 命名空间。
```
using System.Reflection;
```
2. 使用 `Assembly.LoadFrom` 方法加载指定路径下的 DLL。
```
string dllPath = @"C:\MyExternalLibrary.dll";
Assembly externalAssembly = Assembly.LoadFrom(dllPath);
```
3. 使用 `Type.GetType` 方法获取 DLL 中的类型。
```
Type externalType = externalAssembly.GetType("MyExternalLibrary.MyClass");
```
4. 使用 `Activator.CreateInstance` 方法创建类型的实例。
```
object externalObject = Activator.CreateInstance(externalType);
```
5. 使用 `Type.InvokeMember` 方法调用类型的方法。
```
externalType.InvokeMember("MyMethod", BindingFlags.InvokeMethod, null, externalObject, null);
```
以上是在 WPF 中调用指定路径下的 DLL 的基本步骤,可以根据实际情况进行调整。需要注意的是,在使用 `Assembly.LoadFrom` 方法加载 DLL 时,需要确保 DLL 文件存在,并且具有足够的权限被加载。
相关推荐
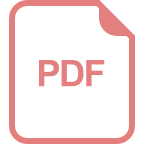












