c语言字符串排序函数
时间: 2024-09-02 19:03:27 浏览: 65
在C语言中,标准库并没有提供直接用于字符串数组排序的内置函数。如果你想对字符串数组进行排序,通常需要借助于其他算法或者第三方库,如qsort或者自定义比较函数传递给stdlib.h的sort函数。
一个常见的做法是使用strcmp()函数来实现冒泡排序、选择排序或快速排序等经典的排序算法。例如,下面是一个简单的冒泡排序示例:
```c
#include <stdio.h>
#include <string.h>
void swap(char **a, char **b) {
char *temp = *a;
*a = *b;
*b = temp;
}
int compare(const void *a, const void *b) {
return strcmp(*(char **)a, *(char **)b);
}
void sort_strings(char *arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (compare(arr + j, arr + j + 1) > 0) {
swap(&arr[j], &arr[j + 1]);
}
}
}
}
int main() {
char *strs[] = {"hello", "world", "C", "Programming"};
int n = sizeof(strs) / sizeof(strs[0]);
printf("Before sorting:\n");
for (int i = 0; i < n; i++) {
printf("%s ", strs[i]);
}
sort_strings(strs, n);
printf("\nAfter sorting:\n");
for (int i = 0; i < n; i++) {
printf("%s ", strs[i]);
}
return 0;
}
```
相关推荐
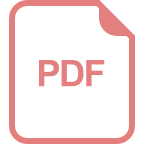
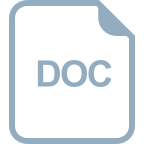
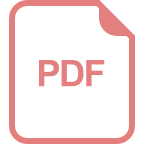














