你的代码不够完整,我无法得到我想要的结果
时间: 2024-09-25 17:02:23 浏览: 29
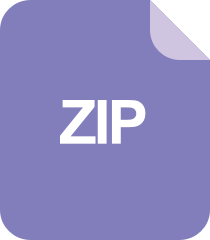
tstem_matlab_tstem函数代码_tstem_tstem函数_

非常抱歉,我理解您可能需要更详细的代码示例才能满足需求。如果您想实现四种常见的边缘检测算法(Roberts、Sobel、Laplacian和Canny),下面是一个完整的C++程序,包括OpenCV库的导入和每个步骤的详细实现:
```cpp
#include <opencv2/opencv.hpp>
#include <vector>
using namespace cv;
std::vector<Mat> detectEdges(const Mat& input, const std::string& algorithm) {
std::vector<Mat> results;
Mat grayImage, temp;
// 转换为灰度图
cvtColor(input, grayImage, COLOR_BGR2GRAY);
if (algorithm == "Roberts") {
// Roberts算子
Mat kernel = (Mat_<double>(2, 2) << 0, -1, -1, 0); // 定义罗伯茨算子
filter2D(grayImage, temp, -1, kernel);
results.push_back(temp);
} else if (algorithm == "Sobel") {
// Sobel算子
Mat sobelX, sobelY;
Scharr(grayImage, sobelX, CV_8U, 1, 0); // 指定方向
Scharr(grayImage, sobelY, CV_8U, 0, 1);
magnitude(sobelX, sobelY, sobelX);
results.push_back(sobelX);
} else if (algorithm == "Laplacian") {
// Laplacian算子
Laplacian(grayImage, temp, CV_8U);
results.push_back(temp);
} else if (algorithm == "Canny") {
// Canny边缘检测
Canny(grayImage, temp, 50, 150, 3);
results.push_back(temp);
} else {
throw std::runtime_error("Invalid edge detection algorithm.");
}
return results;
}
int main() {
try {
// 加载图像
Mat img = imread("path_to_your_image.jpg");
if (img.empty()) {
std::cerr << "Failed to load image." << std::endl;
return -1;
}
// 边缘检测并保存结果
std::vector<std::pair<std::string, Mat>> edgeResults = {
{"Roberts", detectEdges(img, "Roberts")},
{"Sobel", detectEdges(img, "Sobel")},
{"Laplacian", detectEdges(img, "Laplacian")},
{"Canny", detectEdges(img, "Canny")}
};
for (const auto& result : edgeResults) {
std::cout << "Saving result for " << result.first << "..." << std::endl;
imwrite(result.first + "_edge_output.jpg", result.second);
}
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
请将`"path_to_your_image.jpg"`替换为您实际的图像文件路径,并尝试运行此程序。它会生成四种算法的边缘检测结果,并保存到对应的文件夹里。
阅读全文
相关推荐
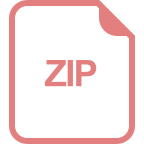
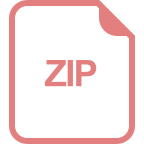
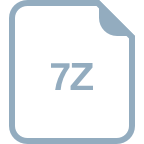
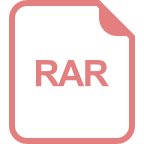
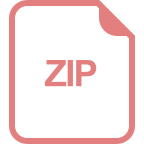
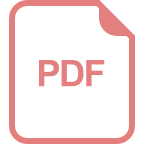
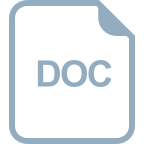
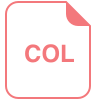
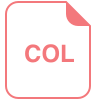
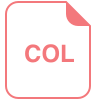
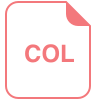
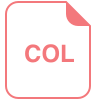
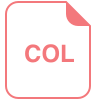
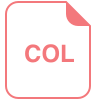
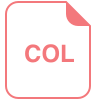
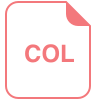
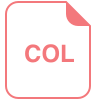
