使用C语言,创建一颗二叉查找树,将以下数据(100 45 67 32 12 4 8 90 87)插入到二叉树中; 2. 查找 32 是否在上面二叉树中,如果属于该树,返回 True;不属于该树,返回 False。 3. 查找二叉树中的最大元素与最小元素。
时间: 2024-12-20 12:22:55 浏览: 5
在C语言中,我们通常使用结构体来表示二叉查找树的节点,并通过递归的方式实现插入、查找和获取最大最小元素的功能。这里是一个简单的示例:
首先,定义一个二叉查找树节点的结构体:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
int data;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
TreeNode* createNode(int value) {
TreeNode* newNode = (TreeNode*)malloc(sizeof(TreeNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
```
然后,可以实现插入操作:
```c
TreeNode* insertIntoBST(TreeNode* root, int value) {
if (root == NULL) {
return createNode(value);
} else if (value < root->data) {
root->left = insertIntoBST(root->left, value);
} else {
root->right = insertIntoBST(root->right, value);
}
return root;
}
// 插入给定的数据
int main() {
int data[] = {100, 45, 67, 32, 12, 4, 8, 90, 87};
TreeNode* root = NULL;
for (int i = 0; i < sizeof(data) / sizeof(data[0]); ++i) {
root = insertIntoBST(root, data[i]);
}
// ... (后续操作)
return 0;
}
```
接下来,我们可以编写查找函数来检查32是否在树中:
```c
bool searchBST(TreeNode* root, int value) {
if (root == NULL || root->data == value) {
return root != NULL;
}
if (value < root->data) {
return searchBST(root->left, value);
} else {
return searchBST(root->right, value);
}
}
```
为了找到最小和最大元素,可以在遍历过程中记录它们:
```c
int findMin(TreeNode* root) {
while (root->left != NULL) {
root = root->left;
}
return root->data;
}
int findMax(TreeNode* root) {
while (root->right != NULL) {
root = root->right;
}
return root->data;
}
```
最后,在`main()`函数中调用这些函数:
```c
int main() {
// ... (之前的操作)
if (searchBST(root, 32)) {
printf("32 is in the tree.\n");
} else {
printf("32 is not in the tree.\n");
}
int minElement = findMin(root);
int maxElement = findMax(root);
printf("Minimum element is %d and maximum element is %d.\n", minElement, maxElement);
return 0;
}
```
阅读全文
相关推荐
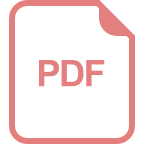
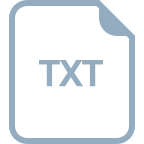
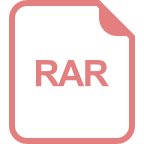









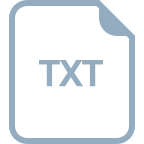
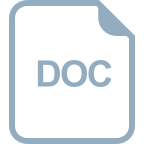
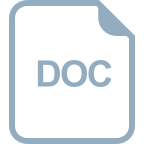

