qt中对实现xml文件增删查改
时间: 2023-09-19 11:10:07 浏览: 47
在 Qt 中,可以使用 QDomDocument 类来实现 XML 文件的增删查改操作。以下是一个示例代码,演示了如何在 XML 文件中执行增删查改操作:
```cpp
#include <QDomDocument>
#include <QFile>
#include <QDebug>
// 增加节点
void addNode(QDomElement& parent, const QString& tagName, const QString& text = "")
{
QDomDocument doc = parent.ownerDocument();
QDomElement element = doc.createElement(tagName);
if (!text.isEmpty())
{
QDomText textNode = doc.createTextNode(text);
element.appendChild(textNode);
}
parent.appendChild(element);
}
// 删除节点
void deleteNode(QDomElement& parent, const QString& tagName)
{
QDomNodeList nodes = parent.elementsByTagName(tagName);
for (int i = nodes.size() - 1; i >= 0; --i)
{
QDomNode node = nodes.at(i);
parent.removeChild(node);
}
}
// 修改节点内容
void modifyNode(QDomElement& parent, const QString& tagName, const QString& newText)
{
QDomNodeList nodes = parent.elementsByTagName(tagName);
for (int i = 0; i < nodes.size(); ++i)
{
QDomNode node = nodes.at(i);
if (node.isElement())
{
QDomElement element = node.toElement();
element.firstChild().setNodeValue(newText);
}
}
}
// 查询节点
void queryNode(const QDomElement& parent, const QString& tagName)
{
QDomNodeList nodes = parent.elementsByTagName(tagName);
for (int i = 0; i < nodes.size(); ++i)
{
QDomNode node = nodes.at(i);
if (node.isElement())
{
QDomElement element = node.toElement();
qDebug() << "标签名:" << element.tagName();
qDebug() << "内容:" << element.text();
}
}
}
int main()
{
// 创建 QDomDocument 对象
QDomDocument doc;
// 加载 XML 文件
QFile file("example.xml");
if (!file.open(QIODevice::ReadOnly | QIODevice::Text))
{
qDebug() << "无法打开文件";
return 1;
}
if (!doc.setContent(&file))
{
file.close();
qDebug() << "无法设置 XML 内容";
return 1;
}
file.close();
// 获取根元素
QDomElement root = doc.documentElement();
// 增加节点
addNode(root, "newNode", "New Node Content");
// 删除节点
deleteNode(root, "oldNode");
// 修改节点内容
modifyNode(root, "existingNode", "Modified Content");
// 查询节点
queryNode(root, "newNode");
// 将修改后的 XML 内容保存到文件
QFile outputFile("output.xml");
if (!outputFile.open(QIODevice::WriteOnly | QIODevice::Text))
{
qDebug() << "无法打开输出文件";
return 1;
}
QTextStream stream(&outputFile);
stream << doc.toString();
outputFile.close();
return 0;
}
```
请注意,您需要将示例代码中的 "example.xml" 替换为您要处理的实际 XML 文件的路径。该示例演示了如何增加节点、删除节点、修改节点内容和查询节点,并将修改后的 XML 内容保存到另一个文件中。
希望这个示例能对您有所帮助!如果您有任何其他问题,请随时提问。
相关推荐
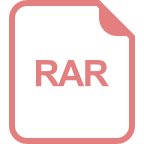
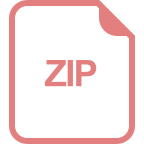














