public static Node createLinkedList(int... arr){ Node head = new Node(); Node tail = null; Node node = null; /* if(arr.length>0) { node = new Node(arr[0]); head.next = node; tail = node; } for(int i=1; i<arr.length; i++) { node = new Node(arr[i]); tail.next = node; tail = node; }*/ for (int i = 0; i < arr.length; i++) { node = new Node(arr[i]); if(i==0){ head.next = node; tail = node; }else{ tail.next = node; tail = node; } } return head; }
时间: 2024-04-13 19:24:46 浏览: 27
这段代码是一个简单的方法用于创建一个单链表。它接受一个整数数组作为参数,并返回一个头节点。
首先,它创建一个头节点 `head`,并初始化尾节点 `tail` 为 `null`。然后,通过循环遍历传入的整数数组,逐个创建节点并将其添加到链表中。
循环中的第一个元素创建的节点将成为头节点的下一个节点,同时也是尾节点。对于数组中的其他元素,它们将被依次添加到尾节点的后面,并更新尾节点为新添加的节点。
最后,返回头节点。这个方法只返回头节点,而不是整个链表,因为头节点可以用来访问链表的其他节点。
相关问题
public static Node createLinkedList(int... arr){ Node head = new Node(); Node tail = null; Node node = null; for (int i = 0; i < arr.length; i++) { node = new Node(arr[i]); if(i==0){ head.next = node; tail = node; }else{ tail.next = node; tail = node; } } return head; }代码优化
这段代码创建一个单链表的方式已经相对简洁,但可以进一步优化。以下是代码的优化版本:
```java
public static Node createLinkedList(int... arr) {
if (arr.length == 0) {
return null;
}
Node head = new Node(arr[0]);
Node tail = head;
for (int i = 1; i < arr.length; i++) {
Node newNode = new Node(arr[i]);
tail.next = newNode;
tail = newNode;
}
return head;
}
```
优化的改进包括:
1. 增加了对空数组的处理,如果传入的整数数组为空,则直接返回 `null`,避免创建一个只有头节点的链表。
2. 在循环中,不再使用 `node` 变量,而是直接使用 `newNode` 创建新节点,并将其连接到尾节点的后面。这样可以减少不必要的变量声明和赋值。
此优化版本与原始代码的功能相同,但更简洁和易读。
for (int i = 0; i < arr.length; i++) { node = new Node(arr[i]); if(i==0){ head.next = node; tail = node; }else{ tail.next = node; tail = node; } }
这段代码使用循环遍历整数数组 `arr`,并根据数组元素创建节点,并将这些节点连接成一个单链表。
首先,它创建一个头节点 `head` 和一个尾节点 `tail`,并将尾节点初始化为 `null`。
然后,通过循环遍历整数数组,使用当前数组元素创建一个新的节点 `node`。
在循环的第一次迭代中(即 `i == 0`),将头节点的 `next` 指针指向新创建的节点 `node`,并将尾节点更新为新节点 `node`。这样,第一个节点就成为了链表的头节点和尾节点。
对于循环的后续迭代(即 `i > 0`),将当前尾节点的 `next` 指针指向新创建的节点 `node`,并将尾节点更新为新节点 `node`。这样,新节点就被添加到了链表的末尾。
最后,返回头节点。
这段代码的功能与之前提供的代码片段相同,只是稍微简化了条件判断的逻辑。
相关推荐
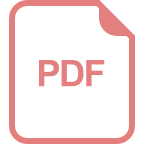
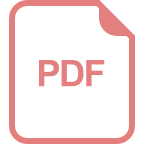
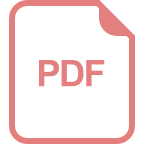













