写一个表单中的上传文件的按钮功能
时间: 2024-09-23 15:11:33 浏览: 18
在Web前端开发中,创建一个表单中的文件上传按钮通常涉及到HTML、CSS以及JavaScript或者相关的文件上传库(比如jQuery、axios或者是原生的FormData API)。这里是一个简单的例子,使用HTML和JavaScript来创建一个基本的文件上传按钮:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<style>
.upload-btn {
padding: 10px;
background-color: #4CAF50;
color: white;
border: none;
cursor: pointer;
width: 200px;
}
</style>
</head>
<body>
<form id="uploadForm">
<label for="fileInput">选择文件:</label>
<input type="file" id="fileInput" name="fileToUpload" accept=".pdf, .doc, .jpg, .png">
<button type="button" class="upload-btn" onclick="submitFile()">上传</button>
<progress id="progressBar" value="0" max="100"></progress>
</form>
<script>
function submitFile() {
const fileInput = document.getElementById('fileInput');
const file = fileInput.files[0];
if (file) {
const formData = new FormData();
formData.append('file', file);
const xhr = new XMLHttpRequest();
xhr.open('POST', '/your-upload-url', true);
xhr.upload.addEventListener('progress', function(e) {
if (e.lengthComputable) {
const percentComplete = (e.loaded / e.total) * 100;
document.getElementById('progressBar').value = percentComplete;
}
});
xhr.onload = function () {
if (xhr.status === 200) {
console.log('文件上传成功:', xhr.responseText);
} else {
console.error('上传失败:', xhr.statusText);
}
};
xhr.send(formData);
} else {
alert('请选择一个文件');
}
}
</script>
</body>
</html>
```
在这个例子中,用户点击“上传”按钮后,会选择文件并将其添加到`FormData`对象中。然后我们通过XMLHttpRequest发送一个POST请求到服务器的指定URL。在上传过程中,进度条会随着文件上传的百分比更新。如果上传成功,响应会被打印出来;如果失败,则会显示错误消息。
注意,你需要将`'/your-upload-url'`替换为实际的文件上传服务器接收请求的地址。同时,对于更复杂的场景,可能还需要考虑错误处理、取消上传等功能,这取决于具体的需求。
相关推荐
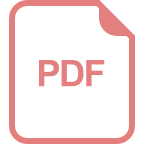
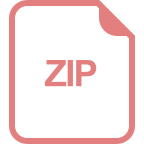
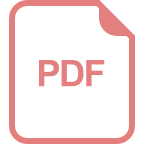














