Vuex.Store的state对象中放一个空的Map对象
时间: 2024-03-10 12:47:00 浏览: 80
在 Vuex.Store 的 state 对象中放一个空的 Map 对象可以这样实现:
```javascript
const store = new Vuex.Store({
state: {
myMap: new Map(),
},
mutations: {
setMap(state, map) {
state.myMap = map;
},
clearMap(state) {
state.myMap.clear();
},
},
actions: {
// ...
},
getters: {
// ...
},
});
```
在上面的代码中,我们在 state 对象中定义了一个名为 "myMap" 的属性,并将其设置为一个空的 Map 对象。我们还定义了两个 mutations,用于设置和清空该 Map 对象。
通过在 state 对象中放一个 Map 对象,我们可以在 Vuex.Store 中存储和管理键值对数据。在 mutations 和 actions 中,我们可以使用 Map 的方法来修改和读取其中的数据。在 getters 中,我们可以将 Map 对象转换为其他形式的数据,以便在组件中使用。
需要注意的是,虽然在 Vuex.Store 中存储 Map 对象是可行的,但在进行数据持久化时可能会遇到一些挑战。因为 Map 对象不能直接序列化为 JSON 格式,所以在将其保存到本地存储或通过网络传输时,需要进行一些额外的处理。
相关问题
vuex 存储Map对象
Vuex是一个专为Vue.js应用程序设计的状态管理模式,它提供了一种集中存储应用状态并协调各组件之间的状态变化的方式。对于存储Map对象,Vuex允许你在store中创建一个mapState、mapGetters、mapActions或mapMutations的对象,这些对象可以用来获取、修改、触发动作等。
例如,你可以这样做:
```javascript
// 定义store中的mapState映射
const state = {
myMap: new Map([
['key1', 'value1'],
['key2', 'value2']
])
}
// 使用mapState获取这个Map对象
export const getters = {
getMyMap: (state) => state.myMap
}
// 然后在组件中通过计算属性或直接访问getter获取Map内容
computed: {
mapValues() {
return this.getMyMap().values()
}
}
```
当需要更新Map对象时,你应该避免直接在store中操作,因为这是不可变的,而是通过commit mutations来进行:
```javascript
export const mutations = {
updateMyMap(state, payload) {
state.myMap = new Map(payload)
}
}
```
然后在actions里调用mutation:
```javascript
export const actions = {
updateMyMap({ commit }, payload) {
commit('updateMyMap', payload)
}
}
```
vuex中的map函数及作用
在 Vue.js 中,Vuex 是一个专门为 Vue.js 应用程序开发的状态管理库。Vuex 将所有组件的状态集中存储在一个单一的地方,称为 state。Vuex 的 state 是响应式的,这意味着当 state 发生变化时,与之相关的组件将自动重新渲染。
为了在组件中使用 Vuex 状态,我们可以使用 mapState 函数。mapState 函数可以接受一个对象或数组作为参数,将 Vuex 中的 state 映射到组件的计算属性中。这样,我们就可以在组件中像使用普通计算属性一样使用 Vuex 的 state。
例如,我们可以将 Vuex 中的 state 映射到组件的计算属性中:
```
import { mapState } from 'vuex'
export default {
computed: {
...mapState({
count: state => state.count
})
}
}
```
上面的代码将 Vuex 中的 count 状态映射到组件的计算属性中。我们可以在组件中像使用普通计算属性一样使用它:
```
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="increment">Increment</button>
</div>
</template>
<script>
import { mapState } from 'vuex'
export default {
computed: {
...mapState({
count: state => state.count
})
},
methods: {
increment() {
this.$store.commit('increment')
}
}
}
</script>
```
在上面的代码中,我们使用了 mapState 函数将 count 状态映射到组件的计算属性中。然后,我们在模板中使用这个计算属性来显示 count 的值。当用户点击“Increment”按钮时,我们调用 Vuex 的 commit 方法来更新 count 状态。由于 count 是响应式的,Vue.js 将自动重新渲染组件来显示新的 count 值。
阅读全文
相关推荐
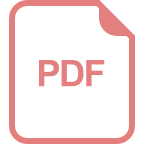
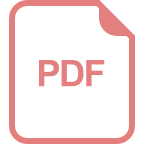
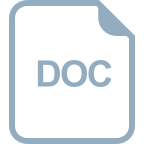
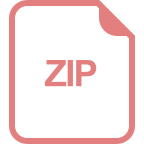
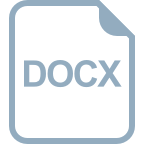
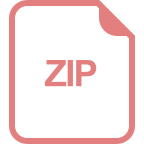
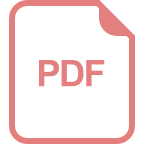
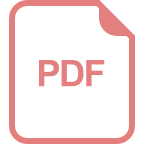
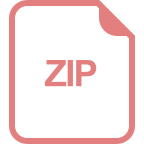
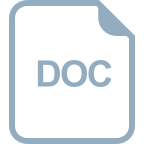
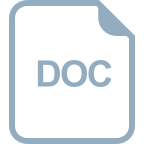
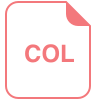
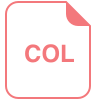
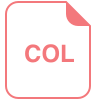


