tensorflow调用VGG16图像预处理的部分
时间: 2023-09-19 07:06:15 浏览: 97
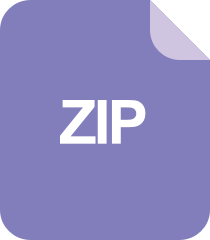
VGG16模型tensorflow实现

以下是使用TensorFlow调用VGG16进行图像预处理的示例代码:
```python
import tensorflow as tf
from tensorflow.keras.applications.vgg16 import preprocess_input
# 加载图像
img = tf.keras.preprocessing.image.load_img('image.jpg', target_size=(224, 224))
# 将图像转换为数组
img_array = tf.keras.preprocessing.image.img_to_array(img)
# 扩充数组的维度,以符合VGG16模型的输入要求
img_array_expanded = tf.expand_dims(img_array, axis=0)
# 进行VGG16的图像预处理
processed_img = preprocess_input(img_array_expanded)
# 打印处理后的图像数组
print(processed_img)
```
在这个示例中,我们首先使用`load_img`函数加载图像,并将其转换为数组。然后,我们使用`expand_dims`函数将数组维度扩充,以符合VGG16模型的输入要求。最后,我们使用`preprocess_input`函数对图像进行预处理,以使其符合VGG16模型的输入要求。预处理后的图像数组将被打印出来。
阅读全文
相关推荐
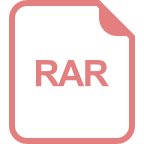
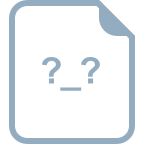
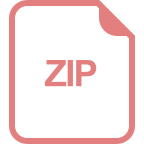
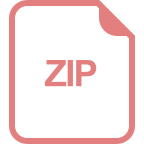
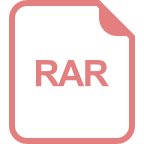
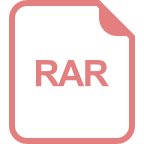
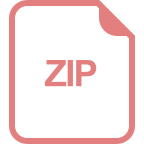
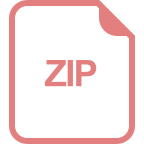
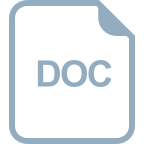
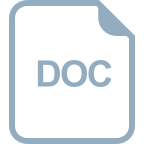
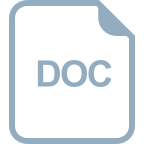
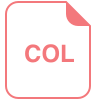
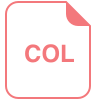
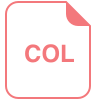
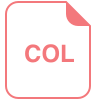
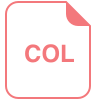
