List<int> aryList1 = new List<int>(); aryList1.AddRange(new int[] { 1, 5, 3 }); List<int> aryList2 = new List<int>(); aryList2.AddRange(new int[] { 9, 7, 3, -2 }); List<int> aryList3 = new List<int>(); aryList3.AddRange(new int[] { 2, 1, 2 }); List<List<int>> aryList4 = new List<List<int>>(); aryList4.Add(aryList1); aryList4.Add(aryList2); aryList4.Add(aryList3); List<int> maxNumbersInLists(List<List<int>> p1) { List<int> result = new List<int>(); for (int i = 0; i < p1.Count; i++) { int max; List<int> temp = p1[i]; for (int j = 1; j < temp.Count; j++) { if (temp[0] < temp[j]) { max = temp[j]; temp[j] = temp[0]; temp[0] = max; } } result.Add(temp[0]); } return result; } for (int i = 0; i < maxNumbersInLists(aryList4).Count; i++) { Console.WriteLine($"List {i + 1} has a maximum of {maxNumbersInLists(aryList4)[i]}."); } 改进
时间: 2024-03-18 21:40:43 浏览: 23
可以对代码进行如下改进:
1. 可以使用 var 关键字来进行变量类型推断,使代码更加简洁。
2. 在找到每个列表的最大值时,可以使用 LINQ 中的 Max() 方法进行查找,避免手动进行循环。
3. 在 for 循环中,maxNumbersInLists(aryList4) 方法被多次调用,可以将其调用结果存储在变量中。
4. 可以使用 foreach 循环来遍历 maxNumbersInLists(aryList4) 方法的结果,使代码更加简洁。
改进后的代码如下:
```
var aryList1 = new List<int> { 1, 5, 3 };
var aryList2 = new List<int> { 9, 7, 3, -2 };
var aryList3 = new List<int> { 2, 1, 2 };
var aryList4 = new List<List<int>> { aryList1, aryList2, aryList3 };
List<int> maxNumbersInLists(List<List<int>> p1)
{
var result = new List<int>();
foreach (var list in p1)
{
result.Add(list.Max());
}
return result;
}
var maxNumbers = maxNumbersInLists(aryList4);
foreach (var maxNumber in maxNumbers)
{
Console.WriteLine($"List {maxNumbers.IndexOf(maxNumber) + 1} has a maximum of {maxNumber}.");
}
```
这样可以使代码更加简洁易读,同时提高了运行效率。
相关推荐
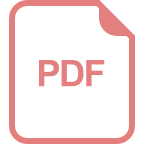
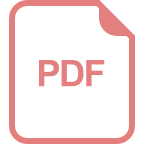
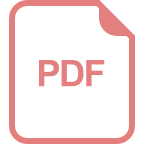














