opencv4.6的情况下,vs2022编写两张图片拼接程序,其中特征点提取使用surf算法
时间: 2023-06-08 22:05:16 浏览: 96
好的,您想了解如何在 VS2022 中使用 OpenCV 4.6 编写图片拼接程序,并使用 SURF 算法提取特征点,对吗?我可以为您提供一些帮助。
首先,您需要确保已经在 VS2022 中安装并配置了 OpenCV 4.6 库。然后,在编写程序之前,您需要了解如何使用 OpenCV 进行图像处理和特征点提取。
首先,您需要使用 OpenCV 中的 imread() 函数加载两张要拼接的图像。然后,您可以使用 SURF 算法(使用 OpenCV 中的 SURF() 函数)在这些图像上提取特征点和描述符。接下来,您可以使用 FLANN 算法(使用 OpenCV 中的 FLANNMatcher() 函数)来匹配这些特征点,并使用 RANSAC 算法(使用 OpenCV 中的 findHomography() 函数)来计算两张图像之间的单应性矩阵,以便将它们拼接在一起。
下面是一些伪代码,可以帮助您了解如何在 VS2022 中使用 OpenCV 4.6 编写两张图片拼接程序,其中特征点提取使用 SURF 算法:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main() {
// Load images
Mat img1 = imread("img1.jpg", IMREAD_GRAYSCALE);
Mat img2 = imread("img2.jpg", IMREAD_GRAYSCALE);
// Extract SURF features and descriptors
Ptr<FeatureDetector> detector = SURF::create();
vector<KeyPoint> keypoints1, keypoints2;
Mat descriptors1, descriptors2;
detector->detect(img1, keypoints1);
detector->detect(img2, keypoints2);
detector->compute(img1, keypoints1, descriptors1);
detector->compute(img2, keypoints2, descriptors2);
// Match SURF features
Ptr<DescriptorMatcher> matcher = FlannBasedMatcher::create();
vector<vector<DMatch>> matches;
matcher->knnMatch(descriptors1, descriptors2, matches, 2);
// Filter matches based on Lowe's ratio test
vector<DMatch> good_matches;
float ratio_thresh = 0.7f;
for (int i = 0; i < matches.size(); i++) {
if (matches[i][0].distance < ratio_thresh * matches[i][1].distance) {
good_matches.push_back(matches[i][0]);
}
}
// Find homography and warp images
vector<Point2f> pts1, pts2;
for (int i = 0; i < good_matches.size(); i++) {
pts1.push_back(keypoints1[good_matches[i].queryIdx].pt);
pts2.push_back(keypoints2[good_matches[i].trainIdx].pt);
}
Mat H = findHomography(pts1, pts2, RANSAC);
Mat result;
warpPerspective(img1, result, H, Size(img1.cols + img2.cols, img1.rows));
img2.copyTo(result(Rect(img1.cols, 0, img2.cols, img2.rows)));
// Save result
imwrite("result.jpg", result);
return 0;
}
```
希望这可以帮助您开始编写自己的图片拼接程序!如果您有任何其他问题,请随时问我。
阅读全文
相关推荐
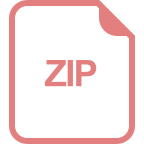
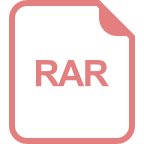
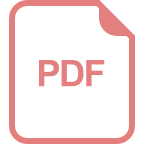
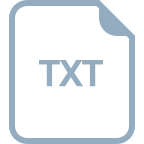
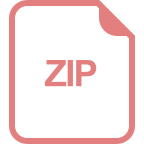
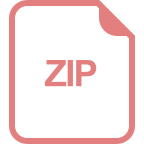
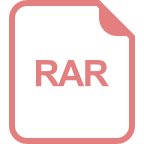
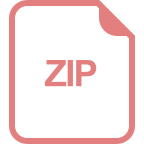
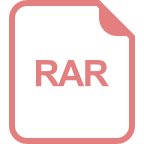
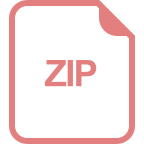
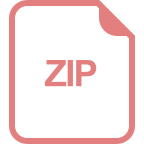
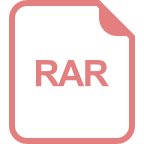
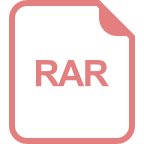
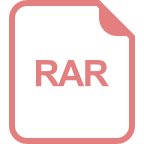
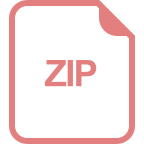
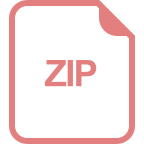
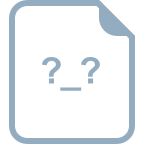