写一段python,实现最近邻耦合网络,展示网络图,并计算平均路径长的和聚类系数
时间: 2023-09-20 10:08:07 浏览: 258
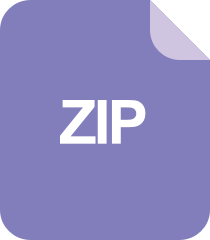
网络的评价路径长度和聚类系数的计算
最近邻耦合网络(nearest-neighbor-coupling network)是一种简单的网络结构,其中每个节点只连接到其相邻的节点。在这里,我们使用 Python 中的 NetworkX 库来实现最近邻耦合网络。
首先,我们需要导入所需的库:
```python
import networkx as nx
import matplotlib.pyplot as plt
```
接下来,我们定义一个函数来创建最近邻耦合网络:
```python
def create_nn_coupling_network(n):
G = nx.Graph()
nodes = range(n)
for i in range(n):
G.add_edge(nodes[i], nodes[(i+1)%n])
G.add_edge(nodes[i], nodes[(i-1)%n])
return G
```
该函数接受一个整数 n,代表节点的数量。它创建一个空的 NetworkX 图,然后将每个节点连接到其相邻的节点。最后,它返回该图。
接下来,我们可以使用该函数创建一个最近邻耦合网络,并绘制它:
```python
G = create_nn_coupling_network(10)
nx.draw(G, with_labels=True)
plt.show()
```
该代码将创建一个包含 10 个节点的最近邻耦合网络,并绘制它。我们可以看到,每个节点只连接到其相邻的节点。
接下来,我们计算该网络的平均路径长度和聚类系数:
```python
avg_shortest_path_length = nx.average_shortest_path_length(G)
clustering_coefficient = nx.average_clustering(G)
print("Average shortest path length:", avg_shortest_path_length)
print("Clustering coefficient:", clustering_coefficient)
```
该代码将计算最近邻耦合网络的平均路径长度和聚类系数,并输出结果。
完整代码如下:
```python
import networkx as nx
import matplotlib.pyplot as plt
def create_nn_coupling_network(n):
G = nx.Graph()
nodes = range(n)
for i in range(n):
G.add_edge(nodes[i], nodes[(i+1)%n])
G.add_edge(nodes[i], nodes[(i-1)%n])
return G
G = create_nn_coupling_network(10)
nx.draw(G, with_labels=True)
plt.show()
avg_shortest_path_length = nx.average_shortest_path_length(G)
clustering_coefficient = nx.average_clustering(G)
print("Average shortest path length:", avg_shortest_path_length)
print("Clustering coefficient:", clustering_coefficient)
```
阅读全文
相关推荐
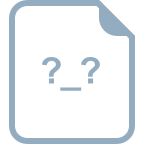
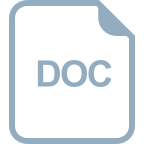
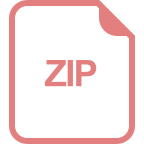
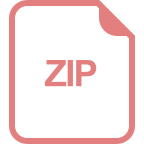
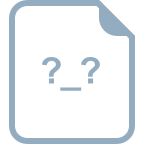
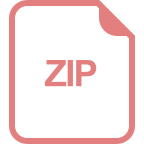
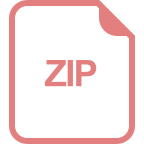
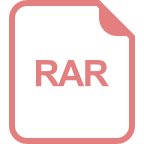
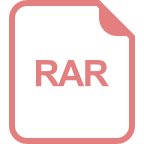
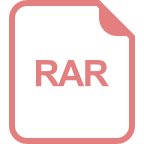
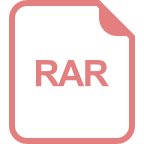
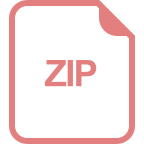
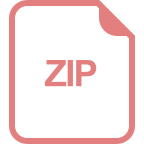