如何使用Python编程来搜索一段英文文本中特定单词的存在?
时间: 2024-10-28 10:06:23 浏览: 30
在Python中,你可以使用内置的`str`对象的`find()`、`index()`或第三方库如`re`(正则表达式)来搜索一段英文文本中的特定单词。以下是基本的示例:
1. 使用`find()`: 这个函数返回目标字符串在原字符串中的起始位置,如果没有找到则返回-1。
```python
def search_word_in_text(text, word):
position = text.find(word)
if position != -1:
return position
else:
return "Word not found."
text = "This is a sample sentence."
word_to_search = "sentence"
print(search_word_in_text(text, word_to_search))
```
2. 使用`index()`: 类似于`find()`,但如果目标单词不存在,会抛出异常,所以需要处理异常。
```python
try:
position = text.index(word_to_search)
except ValueError:
print("Word not found.")
else:
print(position)
```
3. 使用正则表达式库`re`的`search()`函数,可以进行更复杂的模式匹配。
```python
import re
def regex_search(text, word):
match = re.search(r'\b{}\b'.format(word), text) # \b 表示单词边界,防止误匹配
if match:
return match.start()
else:
return None
text = "The quick brown fox jumps over the lazy dog."
word = "fox"
print(regex_search(text, word))
```
阅读全文
相关推荐
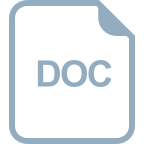
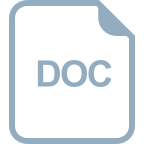
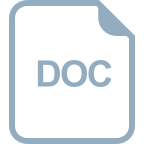
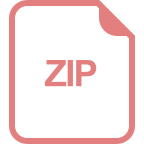
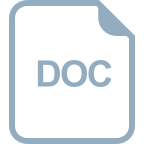
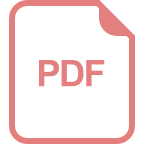
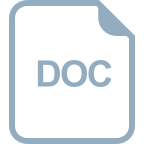
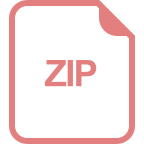
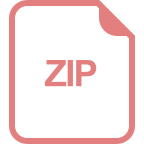
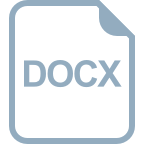
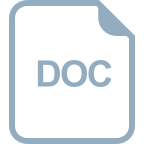
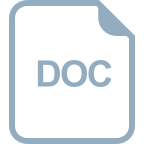
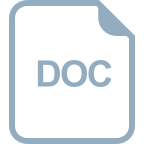
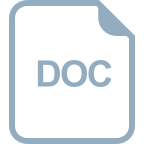
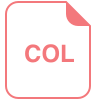
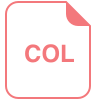
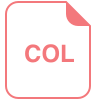
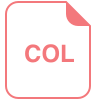
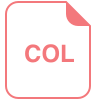