map<string,int>的find函数使用
时间: 2024-05-10 15:21:28 浏览: 3
map<string,int>::find函数用于在map容器中查找指定的键值是否存在,如果存在,则返回该键值对应的迭代器,否则返回map::end迭代器。
例如,以下代码查找map容器中是否存在键值为"apple"的元素:
```c++
#include <iostream>
#include <map>
#include <string>
using namespace std;
int main() {
map<string, int> fruitMap;
fruitMap["apple"] = 10;
fruitMap["banana"] = 20;
map<string, int>::iterator iter = fruitMap.find("apple");
if (iter != fruitMap.end()) {
cout << "apple: " << iter->second << endl;
}
else {
cout << "apple not found" << endl;
}
return 0;
}
```
输出结果为:
```
apple: 10
```
相关问题
unordered_map<int, string> map;
unordered_map<int, string> map是一个无序的关联容器,它将键映射到值。其中键的类型为int,值的类型为string。与map不同的是,unordered_map不会按照键的顺序进行排序,因此它的查找和插入速度更快。可以使用insert()方法或[]运算符向unordered_map中添加数据,也可以使用构造函数进行初始化。同时,unordered_map也提供了其他常用的方法,如erase()、find()等。
map<string,object>拿到double
如果你想从一个`std::map<std::string, object>`中获取一个`double`值,你需要先检查该键是否存在,然后使用`std::get<double>`函数来获取该值。下面是一个示例代码:
```c++
#include <map>
#include <string>
#include <iostream>
#include <variant>
int main() {
std::map<std::string, std::variant<int, double, std::string>> myMap{
{"intVal", 42},
{"dblVal", 3.14159},
{"strVal", "Hello world!"}
};
// Get the double value from the map
if (myMap.find("dblVal") != myMap.end()) {
double dblVal = std::get<double>(myMap["dblVal"]);
std::cout << "The value of dblVal is: " << dblVal << std::endl;
}
return 0;
}
```
在上面的代码中,我们首先定义了一个`std::map<std::string, std::variant<int, double, std::string>>`类型的变量`myMap`,它包含三个键值对,每个键对应一个`int`、一个`double`或一个`std::string`类型的值。
然后我们使用`myMap.find("dblVal")`来检查`myMap`中是否存在键`"dblVal"`,如果存在,我们就使用`std::get<double>(myMap["dblVal"])`来获取该键对应的`double`值。注意,我们使用`std::get`函数来从一个`std::variant`类型中获取具体的值,需要指定要获取的值的类型(在这里是`double`)。如果`myMap["dblVal"]`对应的值不是`double`类型,那么`std::get`函数将会抛出一个`std::bad_variant_access`异常。
相关推荐
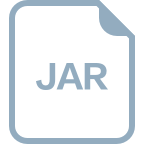
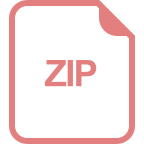
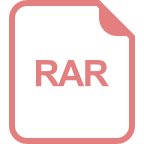













