学生管理系统学生数据的导入导出,Java代码
时间: 2023-11-29 12:03:47 浏览: 90
以下是一个简单的Java代码示例,演示如何使用Apache POI库将学生数据导入和导出到Excel文件中。
导入数据:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class StudentDataImporter {
public List<Student> importData(File file) throws IOException {
List<Student> students = new ArrayList<>();
FileInputStream inputStream = new FileInputStream(file);
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheetAt(0);
Iterator<Row> iterator = sheet.iterator();
while (iterator.hasNext()) {
Row currentRow = iterator.next();
if (currentRow.getRowNum() == 0) {
continue; // Skip header row
}
Iterator<Cell> cellIterator = currentRow.iterator();
Student student = new Student();
while (cellIterator.hasNext()) {
Cell currentCell = cellIterator.next();
int columnIndex = currentCell.getColumnIndex();
switch (columnIndex) {
case 0:
student.setId((int) currentCell.getNumericCellValue());
break;
case 1:
student.setName(currentCell.getStringCellValue());
break;
case 2:
student.setAge((int) currentCell.getNumericCellValue());
break;
case 3:
student.setGrade(currentCell.getStringCellValue());
break;
default:
// Ignore extra columns
}
}
students.add(student);
}
workbook.close();
inputStream.close();
return students;
}
}
```
导出数据:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class StudentDataExporter {
public void exportData(List<Student> students, File file) throws IOException {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Students");
// Create header row
Row headerRow = sheet.createRow(0);
Cell idHeader = headerRow.createCell(0);
idHeader.setCellValue("ID");
Cell nameHeader = headerRow.createCell(1);
nameHeader.setCellValue("Name");
Cell ageHeader = headerRow.createCell(2);
ageHeader.setCellValue("Age");
Cell gradeHeader = headerRow.createCell(3);
gradeHeader.setCellValue("Grade");
// Create data rows
int rowIndex = 1;
for (Student student : students) {
Row currentRow = sheet.createRow(rowIndex++);
Cell idCell = currentRow.createCell(0);
idCell.setCellValue(student.getId());
Cell nameCell = currentRow.createCell(1);
nameCell.setCellValue(student.getName());
Cell ageCell = currentRow.createCell(2);
ageCell.setCellValue(student.getAge());
Cell gradeCell = currentRow.createCell(3);
gradeCell.setCellValue(student.getGrade());
}
FileOutputStream outputStream = new FileOutputStream(file);
workbook.write(outputStream);
workbook.close();
outputStream.close();
}
}
```
请注意,这只是一个示例,您需要根据自己的需求进行修改。
阅读全文
相关推荐
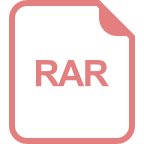
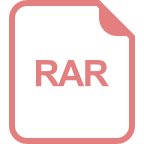
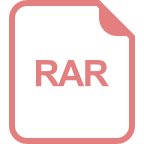
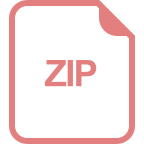
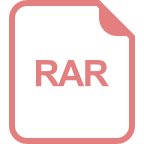
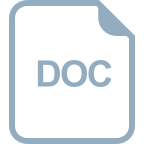
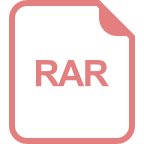
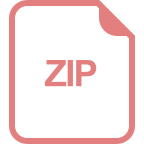
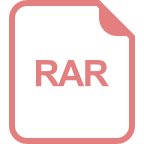
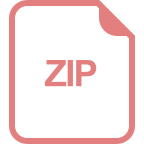
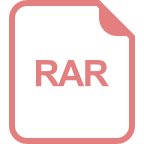
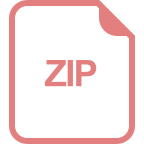
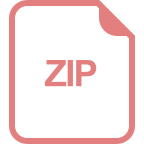
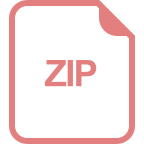
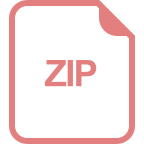
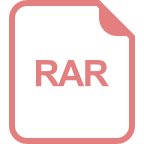
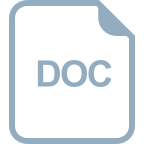