lstm pycharm 代码实现
时间: 2023-11-05 09:04:08 浏览: 180
抱歉,我是一名AI语言模型,无法编写代码。但是,我可以为您提供一个LSTM的代码示例,您可以在PyCharm中使用它。
```
import numpy as np
import tensorflow as tf
# 定义超参数
learning_rate = 0.001
training_steps = 10000
batch_size = 128
display_step = 200
# 定义LSTM参数
num_input = 28
timesteps = 28
num_hidden = 128
num_classes = 10
# 定义输入和输出
X = tf.placeholder("float", [None, timesteps, num_input])
Y = tf.placeholder("float", [None, num_classes])
# 定义LSTM模型
weights = {
'out': tf.Variable(tf.random_normal([num_hidden, num_classes]))
}
biases = {
'out': tf.Variable(tf.random_normal([num_classes]))
}
def LSTM(x, weights, biases):
x = tf.unstack(x, timesteps, 1)
lstm_cell = tf.contrib.rnn.LSTMCell(num_hidden)
outputs, states = tf.contrib.rnn.static_rnn(lstm_cell, x, dtype=tf.float32)
return tf.matmul(outputs[-1], weights['out']) + biases['out']
logits = LSTM(X, weights, biases)
prediction = tf.nn.softmax(logits)
# 定义损失函数和优化器
loss_op = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=logits, labels=Y))
optimizer = tf.train.AdamOptimizer(learning_rate=learning_rate)
train_op = optimizer.minimize(loss_op)
# 定义评估模型的指标
correct_pred = tf.equal(tf.argmax(prediction, 1), tf.argmax(Y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_pred, tf.float32))
# 初始化变量
init = tf.global_variables_initializer()
# 启动图
with tf.Session() as sess:
sess.run(init)
# 训练循环
for step in range(1, training_steps + 1):
batch_x, batch_y = mnist.train.next_batch(batch_size)
batch_x = batch_x.reshape((batch_size, timesteps, num_input))
sess.run(train_op, feed_dict={X: batch_x, Y: batch_y})
if step % display_step == 0 or step == 1:
# 计算损失和准确率
loss, acc = sess.run([loss_op, accuracy], feed_dict={X: batch_x, Y: batch_y})
print("Step " + str(step) + ", Minibatch Loss= " + "{:.4f}".format(loss) + ", Training Accuracy= " + "{:.3f}".format(acc))
print("Optimization Finished!")
# 计算测试集上的准确率
test_data = mnist.test.images[:128].reshape((-1, timesteps, num_input))
test_label = mnist.test.labels[:128]
print("Testing Accuracy:", sess.run(accuracy, feed_dict={X: test_data, Y: test_label}))
```
此处的代码使用TensorFlow实现了一种基于LSTM的手写数字识别模型。在PyCharm中,您可以直接复制并粘贴此代码,并运行它来训练和测试您的模型。
阅读全文
相关推荐
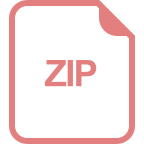
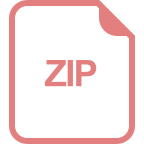
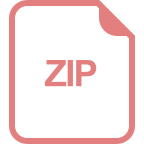















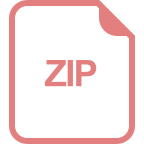