java arrays
时间: 2023-11-13 16:52:24 浏览: 44
在Java中,Arrays类提供了一些常用的方法来操作数组。这些方法包括将数组转换为字符串(toString方法)、数组拷贝与扩容(copyOf方法)、比较两个数组是否相等(equals方法)、填充数组(fill方法)、打印二维数组(deepToString方法)等等。使用这些方法可以方便地进行数组的各种操作。
相关问题
java Arrays
Java arrays are collections of similar data types that are stored in contiguous memory locations. They are used to store a fixed number of elements of the same data type. Arrays in Java are static, which means that their size is fixed and cannot be changed at runtime.
Declaring an array in Java involves specifying the data type of the elements and the size of the array. For example, to declare an array of integers with 5 elements, the syntax would be:
int[] myArray = new int[5];
Arrays can be initialized with values at the time of declaration, like so:
int[] myArray = {1, 2, 3, 4, 5};
Accessing elements of an array is done using their index, which starts at 0. For example, to access the first element of the above array, the syntax would be:
int firstElement = myArray[0];
Arrays can be used in many situations, such as storing data for sorting or searching algorithms, or as a way to represent a grid or matrix of values. They are an important data structure in Java and are used extensively in programming.
java arrays类
Java中的Arrays类是一个工具类,提供了一系列静态方法,用于操作数组。该类提供的方法包括数组排序、数组搜索、数组拷贝、数组填充等。
下面是一些常用的Arrays类方法:
1. sort():对数组进行排序。
2. binarySearch():在数组中查找指定值,并返回其索引值。
3. copyOf():复制一个数组的指定长度。
4. fill():用指定的值填充数组的所有元素。
5. equals():比较两个数组是否相等。
6. asList():将数组转换为List。
下面是一个使用Arrays类的例子,演示了如何对数组进行排序:
```
import java.util.Arrays;
public class ArraysDemo {
public static void main(String[] args) {
int[] arr = {5, 3, 8, 2, 7};
Arrays.sort(arr); // 对数组进行排序
System.out.println(Arrays.toString(arr)); // 输出结果:[2, 3, 5, 7, 8]
}
}
```
需要注意的是,Arrays类中的方法都是静态方法,因此可以直接通过类名调用。
相关推荐
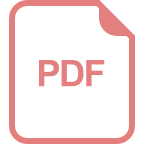
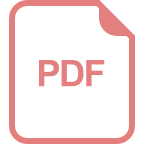
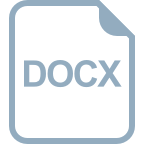
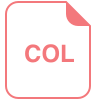
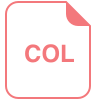










