pywin32 send_message
时间: 2023-07-21 17:29:31 浏览: 116
在pywin32中,可以使用`SendMessage`函数来向指定的窗口或控件发送消息。这个函数的语法为:
```python
SendMessage(hwnd, msg, wparam, lparam)
```
其中,`hwnd`是目标窗口的句柄,`msg`是要发送的消息类型,`wparam`和`lparam`是消息的参数。具体的消息类型和参数可以参考Windows官方文档。
下面是一个示例代码,演示如何向记事本窗口发送`WM_SETTEXT`消息,将文本框中的内容替换为指定的字符串:
```python
import win32gui
import win32con
# 获取记事本窗口句柄
hwnd = win32gui.FindWindow("Notepad", None)
# 获取文本框控件句柄
edit_hwnd = win32gui.FindWindowEx(hwnd, 0, "Edit", None)
# 发送WM_SETTEXT消息,将文本框内容替换为"Hello, world!"
win32gui.SendMessage(edit_hwnd, win32con.WM_SETTEXT, 0, "Hello, world!")
```
这个代码会搜索记事本窗口中的文本框控件,并向它发送`WM_SETTEXT`消息,将文本框的内容替换为"Hello, world!"。你可以根据需要修改目标窗口、控件和消息类型。
相关问题
python postmessage
### 使用 `pywin32` 实现 `PostMessage`
为了在 Python 中实现类似于 JavaScript 的 `postMessage` 功能,在 Windows 平台上通常会借助于 `pywin32` 库来调用底层的 Win32 API 函数。下面是一个具体的例子,展示了如何利用此库发送消息给特定窗口。
#### 安装依赖
首先需要安装 pywin32 扩展包:
```bash
pip install pywin32
```
#### 发送自定义消息到指定窗口
这里展示了一个简单的函数用于向目标窗口句柄 (`hwnd`) 发送带有参数的消息 ID 和附加信息的数据[^2]:
```python
import win32api
def send_custom_message(hwnd, message_id, wparam, lparam):
"""
向指定窗口发送自定义消息
参数:
hwnd (int): 接收消息的目标窗口句柄.
message_id (int): 自定义消息ID.
wparam (any): WPARAM 类型的额外参数.
lparam (any): LPARAM 类型的额外参数.
返回值:
None
"""
try:
win32api.PostMessage(hwnd, message_id, wparam, lparam)
print(f"Message sent successfully to window handle: {hwnd}")
except Exception as e:
print(f"Failed to send message: {e}")
# 示例调用
send_custom_message(123456, 0x400 + 101, "some code", 6 - len(str("some code")))
```
上述代码片段中,`message_id` 被设置成了 WM_USER 加上一个偏移量的形式(即 `WM_USER+101`),这通常是用来区分应用程序特有的消息的一种常见做法;而 `wparam` 和 `lparam` 则分别代表了两个不同的参数传递渠道,可以根据实际需求填充相应的内容。
需要注意的是,这里的 `hwnd` 需要替换为目标应用的实际窗口句柄,可以通过其他工具或编程手段获得。
阅读全文
相关推荐
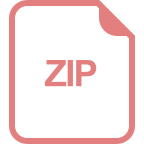















