使用@DS注解实现配置多数据源
时间: 2024-05-01 17:16:43 浏览: 11
@DS注解是Dynamic DataSource Routing的缩写,可以实现动态数据源切换。它可以在方法或类上使用,用于指定使用哪个数据源。下面是一个使用@DS注解实现配置多数据源的示例:
1. 配置多个数据源
```java
@Configuration
public class DataSourceConfig {
@Bean(name = "dataSource1")
@ConfigurationProperties(prefix = "spring.datasource.ds1")
public DataSource dataSource1() {
return DataSourceBuilder.create().build();
}
@Bean(name = "dataSource2")
@ConfigurationProperties(prefix = "spring.datasource.ds2")
public DataSource dataSource2() {
return DataSourceBuilder.create().build();
}
}
```
2. 定义数据源切换注解
```java
@Target({ElementType.METHOD, ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface DS {
String value() default "dataSource1";
}
```
3. 配置数据源切换切面
```java
@Aspect
@Component
public class DynamicDataSourceAspect {
@Pointcut("@annotation(com.example.demo.annotation.DS)")
public void dsPointCut() {
}
@Around("dsPointCut()")
public Object around(ProceedingJoinPoint point) throws Throwable {
MethodSignature signature = (MethodSignature) point.getSignature();
DS ds = signature.getMethod().getAnnotation(DS.class);
if (ds == null) {
DynamicDataSourceContextHolder.setDataSourceKey("dataSource1");
} else {
DynamicDataSourceContextHolder.setDataSourceKey(ds.value());
}
try {
return point.proceed();
} finally {
DynamicDataSourceContextHolder.clearDataSourceKey();
}
}
}
```
4. 在需要使用不同数据源的方法上使用@DS注解
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@DS("dataSource1")
@Override
public User getUserById(int id) {
return userMapper.getUserById(id);
}
@DS("dataSource2")
@Override
public User getUserByUsername(String username) {
return userMapper.getUserByUsername(username);
}
}
```
这样,在调用getUserById方法时会使用dataSource1数据源,调用getUserByUsername方法时会使用dataSource2数据源。
相关推荐
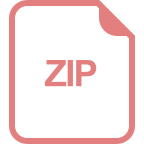
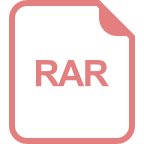














