你现在是c#专家,请帮我用c#语言写一个wpf程序,它需要做到点击按键1,选择读取某一个文件夹下所有dat文件,并在textbox或text block或label中显示文件夹路径。点击按键2,实现该文件夹下所有dat文件的如下转换功能,读取每一行数据,这一行的每一列数据的分隔符从之前的多个空格或逗号或多个tab,全部替换为一个Tab。替换之后覆盖保存原文件。
时间: 2024-03-15 12:45:30 浏览: 102
好的,下面是用C#语言编写的WPF程序的示例代码,可以实现您的需求:
首先,在XAML文件中添加如下布局:
```xml
<Window x:Class="WpfApp1.MainWindow"
...
Title="文件转换工具" Height="250" Width="350">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<TextBlock Text="文件夹路径:" Margin="10" Grid.Row="0"/>
<TextBox x:Name="txtFolderPath" Margin="10" Grid.Row="1"/>
<Button Content="选择文件夹" Margin="10" Grid.Row="2" Click="Button_Click"/>
<Button Content="转换文件" Margin="10" Grid.Row="3" Click="Button_Click_1"/>
</Grid>
</Window>
```
接着,在MainWindow.xaml.cs文件中添加代码实现按键1和按键2的功能:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Windows;
using Microsoft.Win32;
namespace WpfApp1
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void Button_Click(object sender, RoutedEventArgs e)
{
// 弹出文件夹选择对话框
var dlg = new OpenFileDialog();
dlg.InitialDirectory = Environment.GetFolderPath(Environment.SpecialFolder.Desktop);
dlg.Filter = "DAT Files (*.dat)|*.dat";
dlg.Multiselect = false;
dlg.ShowDialog();
// 显示选择的文件夹路径
txtFolderPath.Text = Path.GetDirectoryName(dlg.FileName);
}
private void Button_Click_1(object sender, RoutedEventArgs e)
{
// 获取文件夹路径
var folderPath = txtFolderPath.Text;
// 遍历文件夹下的所有DAT文件
foreach (var filePath in Directory.EnumerateFiles(folderPath, "*.dat"))
{
// 读取文件内容
var lines = File.ReadAllLines(filePath);
// 转换每一行的数据
var newLines = new List<string>();
foreach (var line in lines)
{
var columns = line.Split(new[] { ' ', ',', '\t' }, StringSplitOptions.RemoveEmptyEntries);
var newLine = string.Join("\t", columns);
newLines.Add(newLine);
}
// 覆盖保存原文件
File.WriteAllLines(filePath, newLines, Encoding.UTF8);
}
// 显示转换完成的消息框
MessageBox.Show("文件转换完成!");
}
}
}
```
这样,当用户点击按键1时,会弹出一个文件夹选择对话框,用户选择完成后会将选择的文件夹路径显示在文本框中;当用户点击按键2时,会遍历文件夹下的所有DAT文件,读取每一行数据并进行转换,最后覆盖保存原文件,完成文件转换。
阅读全文
相关推荐













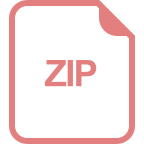

