输入一行字符串,统计字符串中字符的个数,要求用到字符数组
时间: 2024-05-06 09:16:22 浏览: 14
下面是一个 C++ 的例子,可以实现输入一行字符串,统计字符个数并输出:
```cpp
#include <iostream>
using namespace std;
int main() {
const int MAX_LEN = 1000; // 字符串最大长度
char str[MAX_LEN + 1]; // 字符数组,+1 是为了存储字符串结尾的 '\0'
int count = 0; // 字符个数计数器
cout << "请输入一个字符串:";
cin.getline(str, MAX_LEN); // 输入一行字符串,存储到字符数组 str 中
for (int i = 0; str[i] != '\0'; i++) { // 遍历字符数组,直到遇到字符串结尾的 '\0'
count++;
}
cout << "输入的字符串中共有 " << count << " 个字符。" << endl;
return 0;
}
```
这段代码中,我们先定义一个最大长度为 `MAX_LEN` 的字符数组 `str`,然后用 `cin.getline()` 函数从标准输入流中读入一行字符串,并把它存储到字符数组 `str` 中。接着用一个 `for` 循环遍历字符数组,每遍历到一个字符就将计数器 `count` 加 1。最后输出 `count` 的值即可。
相关问题
c语言输入一行字符串,统计字符串中字符的个数
可以使用C语言中的字符串处理函数`strlen()`来实现统计字符串中字符的个数。
示例代码如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
int len;
printf("请输入一个字符串:");
scanf("%s", str);
len = strlen(str);
printf("字符串中字符的个数为:%d\n", len);
return 0;
}
```
在上面的代码中,我们定义了一个字符数组`str`来存储输入的字符串,然后使用`strlen()`函数来计算字符串的长度,最后输出字符的个数。注意,`strlen()`函数计算的是字符串的实际长度,不包括字符串末尾的空字符`\0`。
编写c++,输入一行字符串,统计字符串中字符的个数
以下是使用C++标准库的方法来实现输入一行字符串并统计字符个数的代码:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str;
int count = 0;
cout << "请输入一行字符串:";
getline(cin, str); // 读取一行字符串
for (char c : str) { // 遍历字符串的每个字符
count++;
}
cout << "字符串中字符的个数为:" << count << endl;
return 0;
}
```
在上面的代码中,我们使用 `string` 类型来存储输入的一行字符串,并使用 `getline()` 函数来读取一行字符串(包括空格),然后使用 `for` 循环遍历字符串的每个字符,并将计数器 `count` 加一。最后输出统计结果即可。
相关推荐
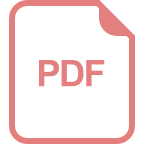












