矩形框生成密度图的代码
时间: 2024-05-07 10:20:46 浏览: 14
以下是生成矩形框密度图的Python代码:
```python
import numpy as np
import cv2
def generate_density_map(image_size, bboxes, sigma=15):
"""
根据矩形框生成密度图
:param image_size: 图像大小
:param bboxes: 矩形框坐标,格式为[x1, y1, x2, y2]
:param sigma: 高斯核大小
:return: 密度图
"""
h, w = image_size
density_map = np.zeros((h, w), dtype=np.float32)
for bbox in bboxes:
x1, y1, x2, y2 = bbox
x1 = int(max(0, min(x1, w - 1)))
y1 = int(max(0, min(y1, h - 1)))
x2 = int(max(0, min(x2, w - 1)))
y2 = int(max(0, min(y2, h - 1)))
# 以矩形框中心点为中心,生成高斯分布
center_x, center_y = (x1 + x2) // 2, (y1 + y2) // 2
radius = sigma * 3
sigma = max(1, sigma)
height, width = y2 - y1, x2 - x1
kernel_size = int(2 * radius + 1)
kernel_size = min(kernel_size, min(height, width))
x = np.arange(kernel_size) - kernel_size // 2
y = np.arange(kernel_size) - kernel_size // 2
xx, yy = np.meshgrid(x, y)
kernel = np.exp(-(xx ** 2 + yy ** 2) / (2 * sigma ** 2))
kernel = kernel / (2 * np.pi * sigma ** 2)
if center_y - radius < 0:
kernel = kernel[int(radius - center_y):]
if center_x - radius < 0:
kernel = kernel[:, int(radius - center_x):]
if center_y + radius >= h:
kernel = kernel[:int(kernel_size - (center_y + radius - h)), :]
if center_x + radius >= w:
kernel = kernel[:, :int(kernel_size - (center_x + radius - w))]
density_map[y1:y2, x1:x2] += kernel
return density_map
```
使用示例:
```python
# 加载图像和矩形框坐标
image = cv2.imread('image.jpg')
bboxes = [[100, 100, 200, 200], [300, 300, 400, 400]]
# 生成密度图
density_map = generate_density_map(image.shape[:2], bboxes)
# 可视化密度图
cv2.imshow('Density Map', density_map)
cv2.waitKey()
```
其中`image_size`参数为图像大小,`bboxes`参数为矩形框坐标,格式为`[x1, y1, x2, y2]`,`sigma`参数为高斯核大小,用于控制密度图的模糊程度。函数返回生成的密度图。
相关推荐
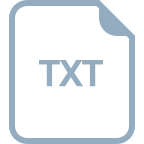














