openssl sm4加解密c语言
时间: 2023-08-06 12:06:07 浏览: 44
以下是使用 OpenSSL 库进行 SM4 加解密的 C 语言代码示例:
```c
#include <openssl/evp.h>
void sm4_encrypt(unsigned char *plaintext, int plaintext_len, unsigned char *key,
unsigned char *iv, unsigned char *ciphertext) {
EVP_CIPHER_CTX *ctx;
int len;
int ciphertext_len;
/* Create and initialize the context */
if (!(ctx = EVP_CIPHER_CTX_new())) {
printf("Error creating SM4 context\n");
return;
}
/* Initialize the encryption operation */
if (1 != EVP_EncryptInit_ex(ctx, EVP_sm4_ecb(), NULL, key, iv)) {
printf("Error initializing SM4 encryption\n");
EVP_CIPHER_CTX_free(ctx);
return;
}
/* Encrypt the plaintext */
if (1 != EVP_EncryptUpdate(ctx, ciphertext, &len, plaintext, plaintext_len)) {
printf("Error encrypting SM4 plaintext\n");
EVP_CIPHER_CTX_free(ctx);
return;
}
ciphertext_len = len;
/* Finalize the encryption */
if (1 != EVP_EncryptFinal_ex(ctx, ciphertext + len, &len)) {
printf("Error finalizing SM4 encryption\n");
EVP_CIPHER_CTX_free(ctx);
return;
}
ciphertext_len += len;
/* Clean up */
EVP_CIPHER_CTX_free(ctx);
}
void sm4_decrypt(unsigned char *ciphertext, int ciphertext_len, unsigned char *key,
unsigned char *iv, unsigned char *plaintext) {
EVP_CIPHER_CTX *ctx;
int len;
int plaintext_len;
/* Create and initialize the context */
if (!(ctx = EVP_CIPHER_CTX_new())) {
printf("Error creating SM4 context\n");
return;
}
/* Initialize the decryption operation */
if (1 != EVP_DecryptInit_ex(ctx, EVP_sm4_ecb(), NULL, key, iv)) {
printf("Error initializing SM4 decryption\n");
EVP_CIPHER_CTX_free(ctx);
return;
}
/* Decrypt the ciphertext */
if (1 != EVP_DecryptUpdate(ctx, plaintext, &len, ciphertext, ciphertext_len)) {
printf("Error decrypting SM4 ciphertext\n");
EVP_CIPHER_CTX_free(ctx);
return;
}
plaintext_len = len;
/* Finalize the decryption */
if (1 != EVP_DecryptFinal_ex(ctx, plaintext + len, &len)) {
printf("Error finalizing SM4 decryption\n");
EVP_CIPHER_CTX_free(ctx);
return;
}
plaintext_len += len;
/* Clean up */
EVP_CIPHER_CTX_free(ctx);
}
```
其中,`sm4_encrypt` 函数用于进行 SM4 加密,`sm4_decrypt` 函数用于进行 SM4 解密。需要传入以下参数:
- `plaintext`:需要加密的明文数据。
- `plaintext_len`:明文数据的长度。
- `key`:加密使用的密钥。
- `iv`:加密使用的初始化向量。
- `ciphertext`:加密后的密文数据(在 `sm4_encrypt` 函数内部生成)。
- `ciphertext_len`:密文数据的长度。
- `plaintext`:需要解密的密文数据。
- `plaintext_len`:密文数据的长度。
- `key`:解密使用的密钥。
- `iv`:解密使用的初始化向量。
- `plaintext`:解密后的明文数据(在 `sm4_decrypt` 函数内部生成)。
请注意,以上代码仅对 ECB 模式进行了 SM4 加解密,如果需要使用其他模式,请更改 `EVP_sm4_ecb()` 函数调用。
相关推荐
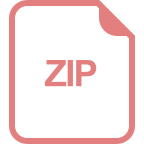














