tensorflow的permute
时间: 2023-08-31 09:05:09 浏览: 64
TensorFlow中的`tf.transpose`可以实现`permute`的功能。`tf.transpose`函数可以对张量的维度进行重新排列,并返回一个新的张量。例如,对于一个形状为`(batch_size, height, width, channels)`的4D张量,我们可以使用`tf.transpose`将其转换为`(batch_size, width, height, channels)`的形状,代码示例如下:
```
import tensorflow as tf
# 创建一个4D张量,形状为(batch_size, height, width, channels)
x = tf.random.normal([32, 256, 256, 3])
# 将张量的维度重新排列,转换为(batch_size, width, height, channels)的形状
x_permuted = tf.transpose(x, perm=[0, 2, 1, 3])
```
在上述代码中,`x`是一个形状为`(32, 256, 256, 3)`的4D张量,`x_permuted`是一个形状为`(32, 256, 256, 3)`的新张量,其中`width`和`height`的位置已经交换。`perm`参数指定了新张量的维度排列方式,`[0, 2, 1, 3]`表示将原来的第1维(`batch_size`)保持不变,将原来的第2维(`height`)和第3维(`width`)交换,最后一维(`channels`)保持不变。
相关问题
如何导入Permute
如果你是使用 PyTorch 框架来进行深度学习的话,可以通过以下代码导入 Permute:
``` python
import torch
x = torch.randn(2, 3, 4, 5) # 生成随机张量
permute_x = x.permute(0, 2, 1, 3) # 对张量进行维度交换
```
这里的 `permute()` 函数可以接受一个整数列表作为参数,用于指定新张量的维度顺序。例如,上述代码中的 `0, 2, 1, 3` 表示将原先张量的第 1 维和第 2 维进行交换。
如果你是使用 TensorFlow 或其他深度学习框架的话,也可以查阅对应的官方文档来寻找 Permute 的用法。
gcn-lstm tensorflow
GCN-LSTM是一种结合了图卷积网络(GCN)和长短时记忆网络(LSTM)的模型,用于处理图数据的时间序列预测问题。下面是使用TensorFlow实现GCN-LSTM的基本步骤:
1.导入必要的库和模块:
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense, LSTM, Dropout, GRU, Bidirectional, Conv1D, MaxPooling1D, Flatten, Reshape, Lambda, Concatenate, Multiply, Add
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.callbacks import EarlyStopping, ModelCheckpoint
from tensorflow.keras import backend as K
```
2.定义GCN层:
```python
class GraphConvolution(tf.keras.layers.Layer):
def __init__(self, output_dim, adj_matrix, **kwargs):
self.output_dim = output_dim
self.adj_matrix = adj_matrix
super(GraphConvolution, self).__init__(**kwargs)
def build(self, input_shape):
self.kernel = self.add_weight(name='kernel',
shape=(input_shape[1], self.output_dim),
initializer='glorot_uniform',
trainable=True)
super(GraphConvolution, self).build(input_shape)
def call(self, x):
adj_matrix = tf.cast(self.adj_matrix, dtype=tf.float32)
output = tf.matmul(adj_matrix, x)
output = tf.matmul(output, self.kernel)
return output
def compute_output_shape(self, input_shape):
return (input_shape[0], self.output_dim)
```
3.定义GCN-LSTM模型:
```python
def gcn_lstm_model(adj_matrix, num_nodes, num_features, num_timesteps_input, num_timesteps_output, num_filters, kernel_size, lstm_units, dropout_rate):
# Input layer
input_layer = Input(shape=(num_timesteps_input, num_nodes, num_features))
# GCN layer
gcn_layer = GraphConvolution(num_filters, adj_matrix)(input_layer)
gcn_layer = Lambda(lambda x: K.permute_dimensions(x, (0, 2, 1, 3)))(gcn_layer)
gcn_layer = Reshape((num_nodes, num_timesteps_input * num_filters))(gcn_layer)
# LSTM layer
lstm_layer = LSTM(lstm_units, return_sequences=True)(gcn_layer)
lstm_layer = Dropout(dropout_rate)(lstm_layer)
# Output layer
output_layer = TimeDistributed(Dense(num_timesteps_output))(lstm_layer)
# Model definition
model = Model(inputs=input_layer, outputs=output_layer)
model.compile(loss='mse', optimizer=Adam(lr=0.001))
return model
```
4.训练模型:
```python
# Define model hyperparameters
num_nodes = 10
num_features = 1
num_timesteps_input = 24
num_timesteps_output = 12
num_filters = 32
kernel_size = 3
lstm_units = 64
dropout_rate = 0.2
batch_size = 32
epochs = 100
# Load data
x_train, y_train, x_val, y_val = load_data()
# Define adjacency matrix
adj_matrix = get_adjacency_matrix(num_nodes)
# Define model
model = gcn_lstm_model(adj_matrix, num_nodes, num_features, num_timesteps_input, num_timesteps_output, num_filters, kernel_size, lstm_units, dropout_rate)
# Train model
early_stopping = EarlyStopping(monitor='val_loss', patience=10)
model_checkpoint = ModelCheckpoint('gcn_lstm.h5', save_best_only=True, save_weights_only=True)
history = model.fit(x_train, y_train, batch_size=batch_size, epochs=epochs, validation_data=(x_val, y_val), callbacks=[early_stopping, model_checkpoint])
```
相关推荐
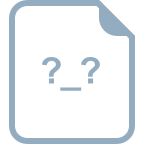













