java抓取网页所有文件
时间: 2023-11-10 15:02:38 浏览: 42
在Java中,要抓取网页上的所有文件,可以使用URL类和URLConnection类来实现。以下是一个基本的实现示例:
```java
import java.io.*;
import java.net.*;
public class WebPageDownloader {
public static void main(String[] args) {
String urlString = "http://example.com"; // 替换为目标网页的URL
try {
URL url = new URL(urlString);
URLConnection connection = url.openConnection();
// 设置User-Agent,模拟浏览器请求
connection.setRequestProperty("User-Agent", "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/83.0.4103.61 Safari/537.36");
// 获取网页的输入流
InputStream inputStream = connection.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
// 逐行读取网页内容
String line;
while ((line = reader.readLine()) != null) {
// 判断是否为文件链接
if (line.contains("<a") && line.contains("href=")) {
int startIndex = line.indexOf("href=\"") + 6;
int endIndex = line.indexOf("\"", startIndex);
String fileUrl = line.substring(startIndex, endIndex);
// 排除非文件链接
if (!fileUrl.contains("#")) {
// 构建文件的URL
URL fileURL = new URL(url, fileUrl);
System.out.println("Downloading file: " + fileURL);
// 下载文件
InputStream fileInputStream = fileURL.openStream();
FileOutputStream fileOutputStream = new FileOutputStream(fileURL.getFile());
byte[] buffer = new byte[1024];
int length;
while ((length = fileInputStream.read(buffer)) != -1) {
fileOutputStream.write(buffer, 0, length);
}
fileInputStream.close();
fileOutputStream.close();
System.out.println("Download completed!");
}
}
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请注意,这只是一个简单的示例,并未考虑到所有可能的情况,比如特殊字符的处理等。在实际使用中,可能还需要根据具体需要进行一些调整和改进。
相关推荐
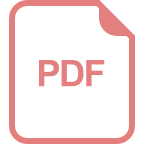














