python实现带图形界面的pdf转word
时间: 2023-06-29 22:11:38 浏览: 101
可以使用Python的PyQt库来实现带图形界面的PDF转Word功能。具体实现步骤如下:
1. 安装PyQt库和pdfminer库。可以使用pip命令进行安装:
```
pip install PyQt5
pip install pdfminer
```
2. 创建一个基于PyQt的GUI界面,包括选择PDF文件、选择输出目录等功能。
3. 使用pdfminer库读取PDF文件,并将内容转换为文本格式。
4. 使用Python的docx库将文本内容写入Word文档中。
下面是一个简单示例代码:
```python
from PyQt5.QtWidgets import QApplication, QFileDialog, QMainWindow, QMessageBox
import sys
import os
import io
import docx
from pdfminer.converter import TextConverter
from pdfminer.pdfinterp import PDFPageInterpreter
from pdfminer.pdfinterp import PDFResourceManager
from pdfminer.pdfpage import PDFPage
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(300, 300, 300, 200)
self.setWindowTitle('PDF转Word')
self.openBtn = QPushButton('打开PDF', self)
self.openBtn.move(50, 50)
self.openBtn.clicked.connect(self.openFile)
self.saveBtn = QPushButton('保存Word', self)
self.saveBtn.move(150, 50)
self.saveBtn.clicked.connect(self.saveFile)
self.show()
def openFile(self):
fname = QFileDialog.getOpenFileName(self, '打开PDF', '', 'PDF files (*.pdf)')[0]
if fname:
self.pdfPath = fname
QMessageBox.information(self, '提示', 'PDF文件已打开!')
def saveFile(self):
if hasattr(self, 'pdfPath'):
fname = QFileDialog.getSaveFileName(self, '保存Word', '', 'Word files (*.docx)')[0]
if fname:
self.wordPath = fname
self.pdf2word()
QMessageBox.information(self, '提示', '转换完成!')
else:
QMessageBox.warning(self, '警告', '请先打开PDF文件!')
def pdf2word(self):
with open(self.pdfPath, 'rb') as pdfFile:
resourceMgr = PDFResourceManager()
outputStr = io.StringIO()
codec = 'utf-8'
converter = TextConverter(resourceMgr, outputStr, codec=codec, laparams=None)
interpreter = PDFPageInterpreter(resourceMgr, converter)
for page in PDFPage.get_pages(pdfFile):
interpreter.process_page(page)
converter.close()
text = outputStr.getvalue()
outputStr.close()
doc = docx.Document()
doc.add_paragraph(text)
doc.save(self.wordPath)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
sys.exit(app.exec_())
```
这段代码实现了一个简单的GUI,包括打开PDF文件、保存Word文件等功能。转换PDF文件为Word文件的具体实现使用了pdfminer库和docx库。注意,在使用pdfminer库时,需要将PDF文件转换为文本格式后才能写入Word文件中。
相关推荐


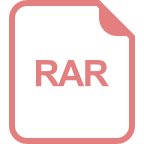
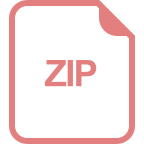
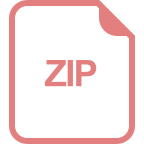
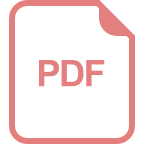
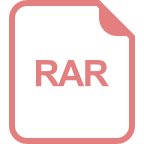
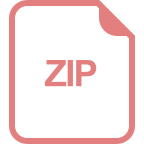
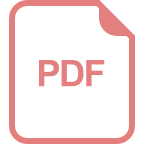
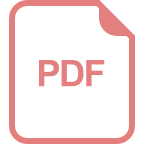
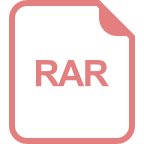
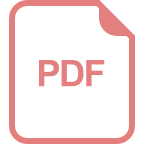
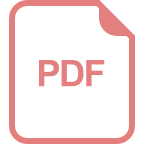
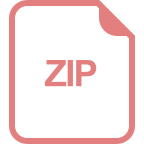
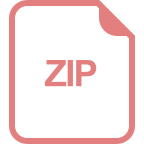