改造Set类,重载+(并集)、(-)差集、*(交集)、<<(输出)、>>(输入)和函数调用操作符(添加一个元素)
时间: 2023-10-17 09:05:08 浏览: 61
以下是改造后的Set类:
```cpp
#include <iostream>
#include <unordered_set>
template<typename T>
class Set {
private:
std::unordered_set<T> set;
public:
Set() {}
Set(std::initializer_list<T> l) {
for (auto& elem : l) {
set.insert(elem);
}
}
Set operator+(const Set& rhs) const {
Set result = *this;
for (auto& elem : rhs.set) {
result.set.insert(elem);
}
return result;
}
Set operator-(const Set& rhs) const {
Set result;
for (auto& elem : set) {
if (!rhs.set.count(elem)) {
result.set.insert(elem);
}
}
return result;
}
Set operator*(const Set& rhs) const {
Set result;
for (auto& elem : set) {
if (rhs.set.count(elem)) {
result.set.insert(elem);
}
}
return result;
}
friend std::ostream& operator<<(std::ostream& os, const Set& s) {
os << "{ ";
for (auto& elem : s.set) {
os << elem << " ";
}
os << "}";
return os;
}
friend std::istream& operator>>(std::istream& is, Set& s) {
T elem;
while (is >> elem) {
s.set.insert(elem);
}
return is;
}
void operator()(const T& elem) {
set.insert(elem);
}
};
```
其中,使用了`std::unordered_set`作为底层数据结构,重载了`operator+`、`operator-`、`operator*`、`operator<<`、`operator>>`和函数调用操作符`operator()`。使用示例如下:
```cpp
int main() {
Set<int> s1 = {1, 2, 3};
Set<int> s2 = {2, 3, 4, 5};
std::cout << "s1 = " << s1 << std::endl;
std::cout << "s2 = " << s2 << std::endl;
std::cout << "s1 + s2 = " << s1 + s2 << std::endl;
std::cout << "s1 - s2 = " << s1 - s2 << std::endl;
std::cout << "s1 * s2 = " << s1 * s2 << std::endl;
Set<std::string> s3;
std::cout << "Enter strings for s3: ";
std::cin >> s3;
std::cout << "s3 = " << s3 << std::endl;
s3("hello");
std::cout << "s3 = " << s3 << std::endl;
return 0;
}
```
输出结果为:
```
s1 = { 1 2 3 }
s2 = { 2 3 4 5 }
s1 + s2 = { 1 2 3 4 5 }
s1 - s2 = { 1 }
s1 * s2 = { 2 3 }
Enter strings for s3: a b c
s3 = { a b c }
s3 = { a b c hello }
```
相关推荐
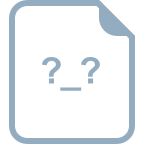
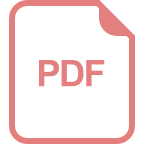
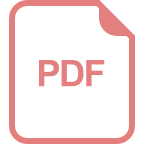
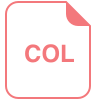
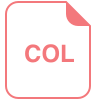












