Java 羽毛球场地预约系统gui界面包含从8:00-20:00的时间,预约按钮,10个场地按钮,取消预约按钮
时间: 2024-03-30 15:38:20 浏览: 43
好的,这是一个关于Java羽毛球场地预约系统GUI界面的问题,界面包含以下元素:
1. 时间:从8:00到20:00,表示一天的时间段。
2. 预约按钮:点击该按钮可以进行场地的预约。
3. 10个场地按钮:表示10个不同的场地,用户可以通过点击这些按钮来选择要预约的场地。
4. 取消预约按钮:点击该按钮可以取消已经预约的场地。
以上就是Java羽毛球场地预约系统GUI界面的元素,用户可以通过这些元素来进行场地的预约和取消预约操作。
相关问题
Java 羽毛球场地预约系统gui界面包含从8:00-20:00的时间,预约按钮,10个场地按钮,取消预约按钮代码
好的,以下是一个简单的Java羽毛球场地预约系统GUI界面的示例代码,包含从8:00到20:00的时间,预约按钮,10个场地按钮和取消预约按钮:
```
import java.awt.*;
import javax.swing.*;
public class BadmintonReservationSystemGUI extends JFrame {
private static final long serialVersionUID = 1L;
private JButton[] courtButtons; // 场地按钮数组
private JButton reserveButton; // 预约按钮
private JButton cancelButton; // 取消预约按钮
public BadmintonReservationSystemGUI() {
super("羽毛球场地预约系统");
setSize(800, 600);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setLayout(new BorderLayout());
// 时间标签面板
JPanel timePanel = new JPanel(new GridLayout(14, 1));
timePanel.add(new JLabel(""));
timePanel.add(new JLabel("8:00"));
timePanel.add(new JLabel("9:00"));
timePanel.add(new JLabel("10:00"));
timePanel.add(new JLabel("11:00"));
timePanel.add(new JLabel("12:00"));
timePanel.add(new JLabel("13:00"));
timePanel.add(new JLabel("14:00"));
timePanel.add(new JLabel("15:00"));
timePanel.add(new JLabel("16:00"));
timePanel.add(new JLabel("17:00"));
timePanel.add(new JLabel("18:00"));
timePanel.add(new JLabel("19:00"));
timePanel.add(new JLabel("20:00"));
// 场地按钮面板
JPanel courtPanel = new JPanel(new GridLayout(14, 11));
courtPanel.add(new JLabel("场地"));
for (int i = 1; i <= 10; i++) {
courtPanel.add(new JLabel(String.valueOf(i)));
}
courtButtons = new JButton[140];
for (int i = 0; i < 140; i++) {
courtButtons[i] = new JButton();
courtPanel.add(courtButtons[i]);
}
// 操作按钮面板
JPanel buttonPanel = new JPanel(new FlowLayout(FlowLayout.CENTER));
reserveButton = new JButton("预约");
cancelButton = new JButton("取消预约");
buttonPanel.add(reserveButton);
buttonPanel.add(cancelButton);
// 添加面板到窗口
add(timePanel, BorderLayout.WEST);
add(courtPanel, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
setVisible(true);
}
public static void main(String[] args) {
new BadmintonReservationSystemGUI();
}
}
```
在这个示例代码中,我们使用了JFrame作为窗口容器,通过GridLayout和FlowLayout来布局界面。其中,时间标签面板和场地按钮面板分别使用了GridLayout布局,预约和取消预约按钮面板使用了FlowLayout布局。
场地按钮使用了JButton类,用于表示10个场地的不同时间段是否已经预约。在按钮点击事件中,我们可以根据按钮的状态来判断用户是否对该时间段的场地进行了预约或取消预约。
以上就是一个简单的Java羽毛球场地预约系统GUI界面的示例代码,你可以参考以上代码来实现你自己的Java羽毛球场地预约系统。
Java 羽毛球场地预约系统gui界面包含从8:00-20:00的时间,室内室外选择,包含日期,预约键,10个场地按钮,取消预约按钮代码
以下是一个简单的 Java GUI 羽毛球场地预约系统界面,包含从8:00-20:00的时间,室内室外选择,包含日期,预约键,10个场地按钮,以及取消预约按钮的代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class BadmintonReservationSystem extends JFrame implements ActionListener {
private JLabel titleLabel, dateLabel, timeLabel, typeLabel, courtLabel;
private JTextField dateTextField;
private JButton reserveButton, cancelButton;
private JRadioButton indoorRadioButton, outdoorRadioButton;
private ButtonGroup typeButtonGroup;
private JButton[] courtButtons;
private int selectedCourt = -1;
public BadmintonReservationSystem() {
// Set up the GUI components
titleLabel = new JLabel("Badminton Court Reservation System");
titleLabel.setFont(new Font("Arial", Font.BOLD, 20));
titleLabel.setHorizontalAlignment(JLabel.CENTER);
dateLabel = new JLabel("Date:");
dateTextField = new JTextField(10);
timeLabel = new JLabel("Time:");
JPanel timePanel = new JPanel(new GridLayout(2, 6));
for (int i = 8; i <= 20; i++) {
timePanel.add(new JLabel(i + ":00"));
}
typeLabel = new JLabel("Type:");
indoorRadioButton = new JRadioButton("Indoor", true);
outdoorRadioButton = new JRadioButton("Outdoor");
typeButtonGroup = new ButtonGroup();
typeButtonGroup.add(indoorRadioButton);
typeButtonGroup.add(outdoorRadioButton);
courtLabel = new JLabel("Court:");
courtButtons = new JButton[10];
for (int i = 0; i < 10; i++) {
courtButtons[i] = new JButton("Court " + (i + 1));
courtButtons[i].addActionListener(this);
}
reserveButton = new JButton("Reserve");
reserveButton.addActionListener(this);
cancelButton = new JButton("Cancel Reservation");
cancelButton.addActionListener(this);
// Add the components to the JFrame
JPanel mainPanel = new JPanel(new GridLayout(6, 1));
mainPanel.add(titleLabel);
JPanel datePanel = new JPanel(new FlowLayout());
datePanel.add(dateLabel);
datePanel.add(dateTextField);
mainPanel.add(datePanel);
mainPanel.add(timeLabel);
mainPanel.add(timePanel);
JPanel typePanel = new JPanel(new FlowLayout());
typePanel.add(typeLabel);
typePanel.add(indoorRadioButton);
typePanel.add(outdoorRadioButton);
mainPanel.add(typePanel);
JPanel courtPanel = new JPanel(new FlowLayout());
courtPanel.add(courtLabel);
for (int i = 0; i < 10; i++) {
courtPanel.add(courtButtons[i]);
}
mainPanel.add(courtPanel);
JPanel buttonPanel = new JPanel(new FlowLayout());
buttonPanel.add(reserveButton);
buttonPanel.add(cancelButton);
mainPanel.add(buttonPanel);
add(mainPanel);
// Set up the JFrame
setTitle("Badminton Court Reservation System");
setSize(600, 400);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == reserveButton) {
String date = dateTextField.getText();
String time = null;
for (int i = 8; i <= 20; i++) {
if (courtButtons[i - 8].isEnabled()) {
time = i + ":00";
}
}
String type = indoorRadioButton.isSelected() ? "Indoor" : "Outdoor";
String court = "Court " + (selectedCourt + 1);
if (date != null && time != null && type != null && court != null) {
JOptionPane.showMessageDialog(null, "Reservation successful!");
} else {
JOptionPane.showMessageDialog(null, "Please select a court.");
}
} else if (e.getSource() == cancelButton) {
for (int i = 0; i < 10; i++) {
courtButtons[i].setEnabled(true);
}
selectedCourt = -1;
} else {
for (int i = 0; i < 10; i++) {
if (e.getSource() == courtButtons[i]) {
selectedCourt = i;
courtButtons[i].setEnabled(false);
} else {
courtButtons[i].setEnabled(true);
}
}
}
}
public static void main(String[] args) {
new BadmintonReservationSystem();
}
}
```
注意:这只是一个简单的实现,你需要根据你的项目需求进行修改和完善。
阅读全文
相关推荐



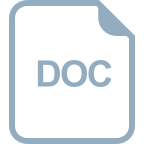

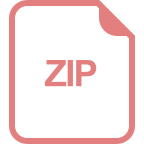
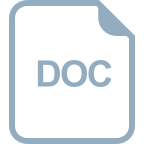
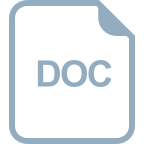
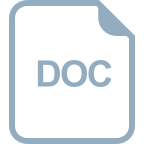
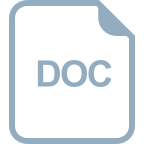
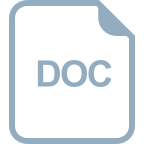