如何建立语言模型?请给出详细步骤和相关代码
时间: 2023-06-06 18:07:56 浏览: 173
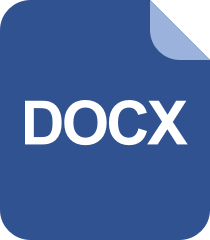
WizardCoder代码大语言模型论文研读+原理解析
建立语言模型的步骤大致如下:
1. 收集语料库:搜索相关数据集或创建自己的语料库。
2. 预处理:对语料进行清洗、分词和标记。
3. 构建词汇表:建立词汇表和每个词对应的 ID。
4. 训练模型:使用具有特定架构的神经网络对语料进行训练。
5. 应用模型:使用模型生成新的文本。
相关 Python 代码:
1. 收集语料库:
```
import urllib.request
url = "https://url-to-corpus.com/corpus.txt"
urllib.request.urlretrieve(url, "corpus.txt")
```
2. 预处理:
```
import re
def clean_text(text):
text = re.sub(r'[^\w\s]','',text)
text = re.sub(r'\d+','',text)
text = text.lower()
text = text.strip()
return text
def tokenize_text(text):
tokens = text.split()
return tokens
def prepare_corpus(filepath):
with open(filepath, 'r', encoding='utf-8') as f:
text = f.read()
text = clean_text(text)
tokens = tokenize_text(text)
return tokens
```
3. 构建词汇表:
```
import collections
def build_vocab(tokens):
word_counts = collections.Counter(tokens)
vocabulary_inv = [x[0] for x in word_counts.most_common()]
vocabulary = {x: i for i, x in enumerate(vocabulary_inv)}
return [vocabulary, vocabulary_inv]
```
4. 训练模型:
```
import tensorflow as tf
def build_model(vocab_size, embedding_dim, rnn_units):
model = tf.keras.Sequential([
tf.keras.layers.Embedding(vocab_size, embedding_dim),
tf.keras.layers.LSTM(rnn_units, return_sequences=True),
tf.keras.layers.Dense(vocab_size)
])
return model
def compile_model(model, learning_rate):
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=learning_rate),
loss=tf.losses.SparseCategoricalCrossentropy(from_logits=True))
def train_model(model, input_data, epochs, batch_size, checkpoint_dir):
checkpoint_callback = tf.keras.callbacks.ModelCheckpoint(
filepath=os.path.join(checkpoint_dir,'ckpt_{epoch}'),save_weights_only=True)
model.fit(input_data, epochs=epochs, callbacks=[checkpoint_callback], batch_size=batch_size)
```
5. 应用模型:
```
def generate_text(model, start_string, vocabulary_inv, num_generate):
input_eval = [vocabulary[word] for word in start_string.split()]
input_eval = tf.expand_dims(input_eval, 0)
text_generated = []
temperature = 0.5
model.reset_states()
for i in range(num_generate):
predictions = model(input_eval)
predictions = tf.squeeze(predictions, 0)
predictions = predictions / temperature
predicted_id = tf.random.categorical(predictions, num_samples=1)[-1,0].numpy()
input_eval = tf.expand_dims([predicted_id], 0)
text_generated.append(vocabulary_inv[predicted_id])
return (start_string + ' '.join(text_generated))
```
阅读全文
相关推荐
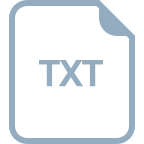
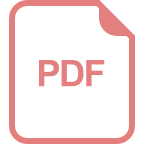














