帮我用python写一段收发邮件的代码,收邮件为检索某一标题的邮件是否出现,未出现返回False,出现返回(True,邮件内容)的代码并封装
时间: 2024-03-25 07:36:24 浏览: 80
好的,以下是收发邮件的代码,其中包括一个函数 `search_email`,用于搜索包含特定标题的邮件。如果找到了这样的邮件,则返回一个元组 `(True, 邮件内容)`,否则返回 `False`。
```python
import imaplib
import email
from email.header import decode_header
import smtplib
from email.mime.text import MIMEText
class EmailClient:
def __init__(self, imap_server, smtp_server, username, password):
self.imap_server = imap_server
self.smtp_server = smtp_server
self.username = username
self.password = password
def search_email(self, search_subject):
# log in to the IMAP server
imap = imaplib.IMAP4_SSL(self.imap_server)
imap.login(self.username, self.password)
imap.select('INBOX')
# search for emails with the given subject
result, data = imap.search(None, f'SUBJECT "{search_subject}"')
if result != 'OK':
return False
# get the latest email with the given subject
latest_email_id = data[0].split()[-1]
result, data = imap.fetch(latest_email_id, "(RFC822)")
if result != 'OK':
return False
# parse the email content
email_message = email.message_from_bytes(data[0][1])
subject = decode_header(email_message["Subject"])[0][0]
body = email_message.get_payload(decode=True).decode()
# log out of the IMAP server
imap.close()
imap.logout()
return True, body
def send_email(self, to_email, subject, body):
# log in to the SMTP server
smtp = smtplib.SMTP_SSL(self.smtp_server)
smtp.login(self.username, self.password)
# create the email message
message = MIMEText(body)
message['From'] = self.username
message['To'] = to_email
message['Subject'] = subject
# send the email
smtp.sendmail(self.username, [to_email], message.as_string())
# log out of the SMTP server
smtp.quit()
if __name__ == '__main__':
# create an email client object
email_client = EmailClient(
'imap.example.com',
'smtp.example.com',
'your_username',
'your_password'
)
# search for an email with the given subject
search_subject = 'Test Email'
result = email_client.search_email(search_subject)
if result:
print(f'Found email with subject "{search_subject}":\n{result[1]}')
else:
print(f'No email found with subject "{search_subject}"')
```
你可以将 `EmailClient` 类实例化,然后使用其中的 `search_email` 和 `send_email` 方法来搜索邮件和发送邮件。例如,下面的代码将创建一个 `EmailClient` 对象并向 `to_email` 发送一封主题为 `subject`,正文为 `body` 的电子邮件:
```python
email_client = EmailClient(imap_server, smtp_server, username, password)
email_client.send_email(to_email, subject, body)
```
希望这可以帮到你!
阅读全文
相关推荐
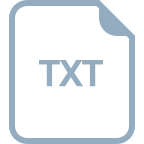
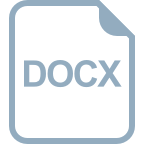
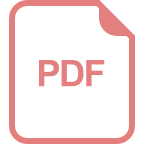
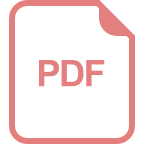
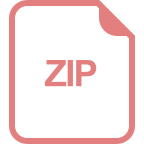
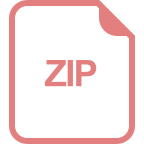
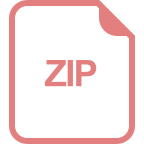
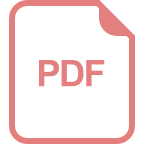
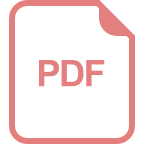
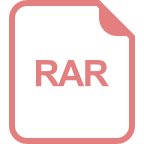
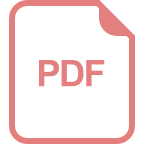
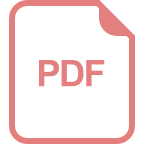
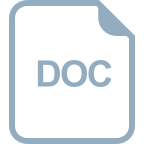