Qtcpsocket 实现客户端与客户端之间的传输文件
时间: 2023-10-02 13:05:33 浏览: 106
要在Qt中实现客户端与客户端之间的文件传输,可以使用QTcpSocket类。以下是一个简单的示例,用于在客户端之间传输文件。
首先,需要创建一个服务器,以便客户端可以连接到它并进行文件传输。以下是一个简单的示例:
```cpp
QTcpServer *server = new QTcpServer(this);
connect(server, SIGNAL(newConnection()), this, SLOT(newConnection()));
if(!server->listen(QHostAddress::Any, 1234)) {
qDebug() << "Server could not start!";
} else {
qDebug() << "Server started!";
}
void MyClass::newConnection(){
QTcpSocket *socket = server->nextPendingConnection();
connect(socket, SIGNAL(readyRead()), this, SLOT(readyRead()));
connect(socket, SIGNAL(disconnected()), socket, SLOT(deleteLater()));
}
void MyClass::readyRead(){
QTcpSocket *socket = qobject_cast<QTcpSocket *>(sender());
if(socket->bytesAvailable() > 0) {
QByteArray data = socket->readAll();
// Do something with the data
}
}
```
在上面的示例中,服务器将侦听端口1234,并在有新连接时调用newConnection()函数。在newConnection()函数中,我们创建一个新的QTcpSocket对象,并将其连接到readyRead()和disconnected()信号。在readyRead()函数中,我们读取从客户端发送的任何数据。
现在,我们需要创建一个客户端,以便它可以连接到服务器并发送文件。以下是一个简单的示例:
```cpp
QTcpSocket *socket = new QTcpSocket(this);
connect(socket, SIGNAL(connected()), this, SLOT(connected()));
connect(socket, SIGNAL(readyRead()), this, SLOT(readyRead()));
connect(socket, SIGNAL(disconnected()), this, SLOT(disconnected()));
socket->connectToHost("localhost", 1234);
void MyClass::connected(){
QFile file("test.txt");
if(!file.open(QIODevice::ReadOnly)) {
qDebug() << "Could not open file!";
return;
}
QByteArray data = file.readAll();
socket->write(data);
file.close();
}
void MyClass::readyRead(){
if(socket->bytesAvailable() > 0) {
QByteArray data = socket->readAll();
// Do something with the data
}
}
void MyClass::disconnected(){
qDebug() << "Disconnected!";
}
```
在上面的示例中,我们创建了一个QTcpSocket对象,并将其连接到connected()、readyRead()和disconnected()信号。在connected()函数中,我们打开要发送的文件,读取其内容,并使用QTcpSocket的write()函数将其发送到服务器。在readyRead()函数中,我们读取从服务器发送的任何数据。在disconnected()函数中,我们打印出已断开连接的消息。
这是一种非常基本的方法,可用于在客户端之间传输文件。当然,你可以根据需要添加更多功能,例如错误处理和进度跟踪等。
相关推荐
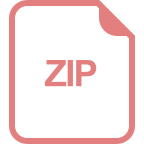
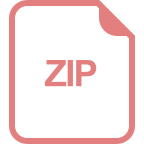














