编写一段c语言程序,使用系统调用 fork( )创建两个子进程,再用系统 调用 signal( )让父进程捕捉组合键的中断信号(即按 ctrl+c 键),当 捕捉到中断信号后,父进程用系统调用 kill( )向两个子进程发出信号, 子进程捕捉到父进程发来的信号后,分别输出下列信息后终止。 的问题反馈
时间: 2024-02-18 09:01:09 浏览: 64
以下是使用 C 语言编写的程序,通过 `fork()` 系统调用创建两个子进程,并使用 `signal()` 系统调用让父进程捕捉中断信号。当捕捉到中断信号后,父进程向两个子进程发送信号,子进程捕捉到信号后输出信息并终止。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
void childProcess(int num) {
printf("Child process %d received signal from parent\n", num);
exit(0);
}
int main() {
pid_t pid1, pid2;
pid1 = fork();
if (pid1 < 0) {
perror("Error creating first child process");
exit(1);
} else if (pid1 == 0) {
// Child process 1
signal(SIGINT, SIG_IGN);
printf("Child process 1 running\n");
while (1) {
// Do some work
}
exit(0);
}
pid2 = fork();
if (pid2 < 0) {
perror("Error creating second child process");
exit(1);
} else if (pid2 == 0) {
// Child process 2
signal(SIGINT, SIG_IGN);
printf("Child process 2 running\n");
while (1) {
// Do some work
}
exit(0);
}
// Parent process
printf("Parent process running\n");
signal(SIGINT, SIG_IGN);
printf("Press Ctrl+C to send signal to child processes\n");
while (1) {
// Wait for signal
}
// Signal received
printf("Parent process received signal\n");
kill(pid1, SIGUSR1);
kill(pid2, SIGUSR1);
// Wait for child processes to terminate
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
return 0;
}
```
在上述程序中,子进程在运行时忽略中断信号,而父进程在运行时等待中断信号。当父进程捕捉到中断信号后,会向两个子进程发送 `SIGUSR1` 信号,子进程捕捉到该信号后会输出信息并终止。在程序中,`waitpid()` 函数用于等待子进程终止。
阅读全文
相关推荐
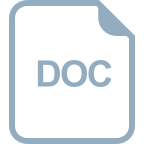
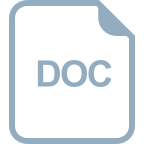
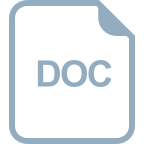
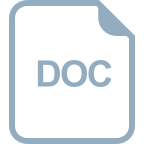
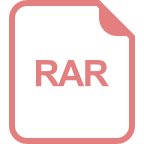
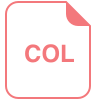
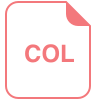
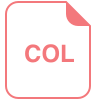
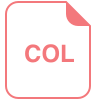
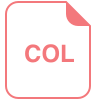
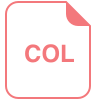
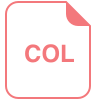
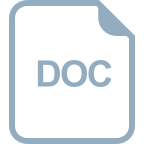
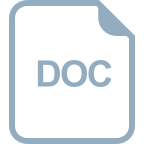