y10 = np.zeros((m,num_labels))
时间: 2023-06-13 14:06:09 浏览: 51
这行代码是用来创建一个名为y10的零矩阵,其大小为(m, num_labels),其中m是样本数量,num_labels是标签数量。它通常用于多分类问题中,其中每个样本可以属于多个类别中的一个或多个,因此需要将每个标签表示为一个二进制数组,其中每个元素表示该样本是否属于该标签。该行代码创建了一个初始全部为零的矩阵,用于存储每个样本属于每个标签的二进制表示。
相关问题
in_features = train_features.shape[1] def train(model, train_features, train_labels, test_features, test_labels, num_epochs, learning_rate, weight_decay, batch_size): train_ls, test_ls = [], [] theta = np.zeros((in_features, 1)) best_theta = np.zeros((in_features, 1)) best_loss = np.inf for epoch in range(num_epochs): train_iter = data_iter(batch_size, train_features, train_labels) for X, y in train_iter: theta=gradientDescent(X, y, theta, learning_rate, weight_decay) train_ls.append(log_rmse(model, train_features, train_labels, theta, len(train_labels)))帮我加个注释
# in_features表示输入特征的数量
in_features = train_features.shape[1]
# 定义训练函数,接受模型、训练数据、测试数据、超参数等作为输入
def train(model, train_features, train_labels, test_features, test_labels,
num_epochs, learning_rate, weight_decay, batch_size):
# 初始化训练误差和测试误差列表
train_ls, test_ls = [], []
# 初始化模型参数theta(权重)
theta = np.zeros((in_features, 1))
# 初始化最佳模型参数和最小测试误差
best_theta = np.zeros((in_features, 1))
best_loss = np.inf
# 循环迭代训练num_epochs次
for epoch in range(num_epochs):
# 随机生成batch_size大小的数据批次,用于训练
train_iter = data_iter(batch_size, train_features, train_labels)
# 遍历数据批次,计算梯度并更新模型参数theta
for X, y in train_iter:
theta=gradientDescent(X, y, theta, learning_rate, weight_decay)
# 计算每轮迭代后的训练误差和测试误差,并存入对应的列表中
train_ls.append(log_rmse(model, train_features, train_labels, theta, len(train_labels)))
test_ls.append(log_rmse(model, test_features, test_labels, theta, len(test_labels)))
# 如果当前模型参数对应的测试误差比历史最小值更小,则更新最佳模型参数和最小测试误差
if test_ls[-1] < best_loss:
best_theta = theta
best_loss = test_ls[-1]
# 返回最佳模型参数和训练误差、测试误差列表
return best_theta, train_ls, test_ls
LDAM损失函数pytorch代码如下:class LDAMLoss(nn.Module): def init(self, cls_num_list, max_m=0.5, weight=None, s=30): super(LDAMLoss, self).init() m_list = 1.0 / np.sqrt(np.sqrt(cls_num_list)) m_list = m_list * (max_m / np.max(m_list)) m_list = torch.cuda.FloatTensor(m_list) self.m_list = m_list assert s > 0 self.s = s if weight is not None: weight = torch.FloatTensor(weight).cuda() self.weight = weight self.cls_num_list = cls_num_list def forward(self, x, target): index = torch.zeros_like(x, dtype=torch.uint8) index_float = index.type(torch.cuda.FloatTensor) batch_m = torch.matmul(self.m_list[None, :], index_float.transpose(1,0)) # 0,1 batch_m = batch_m.view((16, 1)) # size=(batch_size, 1) (-1,1) x_m = x - batch_m output = torch.where(index, x_m, x) if self.weight is not None: output = output * self.weight[None, :] target = torch.flatten(target) # 将 target 转换成 1D Tensor logit = output * self.s return F.cross_entropy(logit, target, weight=self.weight) 模型部分参数如下:# 设置全局参数 model_lr = 1e-5 BATCH_SIZE = 16 EPOCHS = 50 DEVICE = torch.device('cuda:0' if torch.cuda.is_available() else 'cpu') use_amp = True use_dp = True classes = 7 resume = None CLIP_GRAD = 5.0 Best_ACC = 0 #记录最高得分 use_ema=True model_ema_decay=0.9998 start_epoch=1 seed=1 seed_everything(seed) # 数据增强 mixup mixup_fn = Mixup( mixup_alpha=0.8, cutmix_alpha=1.0, cutmix_minmax=None, prob=0.1, switch_prob=0.5, mode='batch', label_smoothing=0.1, num_classes=classes) # 读取数据集 dataset_train = datasets.ImageFolder('/home/adminis/hpy/ConvNextV2_Demo/RAF-DB/RAF/train', transform=transform) dataset_test = datasets.ImageFolder("/home/adminis/hpy/ConvNextV2_Demo/RAF-DB/RAF/valid", transform=transform_test) 帮我用pytorch实现模型在模型训练中使用LDAM损失函数
好的,下面是使用LDAM损失函数训练模型的代码:
```python
import torch.optim as optim
from torch.optim.lr_scheduler import CosineAnnealingLR
from torch.utils.data import DataLoader
# 定义LDAM损失函数
class LDAMLoss(nn.Module):
def __init__(self, cls_num_list, max_m=0.5, weight=None, s=30):
super(LDAMLoss, self).__init__()
m_list = 1.0 / np.sqrt(np.sqrt(cls_num_list))
m_list = m_list * (max_m / np.max(m_list))
m_list = torch.cuda.FloatTensor(m_list)
self.m_list = m_list
assert s > 0
self.s = s
if weight is not None:
weight = torch.FloatTensor(weight).cuda()
self.weight = weight
self.cls_num_list = cls_num_list
def forward(self, x, target):
index = torch.zeros_like(x, dtype=torch.uint8)
index_float = index.type(torch.cuda.FloatTensor)
batch_m = torch.matmul(self.m_list[None, :], index_float.transpose(1,0)) # 0,1
batch_m = batch_m.view((x.size(0), 1)) # size=(batch_size, 1)
x_m = x - batch_m
output = torch.where(index, x_m, x)
if self.weight is not None:
output = output * self.weight[None, :]
target = torch.flatten(target) # 将 target 转换成 1D Tensor
logit = output * self.s
return F.cross_entropy(logit, target, weight=self.weight)
# 定义模型
model = models.resnet18(pretrained=True)
num_ftrs = model.fc.in_features
model.fc = nn.Linear(num_ftrs, classes)
model.to(DEVICE)
# 定义优化器和学习率调整器
optimizer = optim.Adam(model.parameters(), lr=model_lr)
scheduler = CosineAnnealingLR(optimizer, T_max=EPOCHS, eta_min=1e-6)
# 定义LDAM损失函数
cls_num_list = [len(dataset_train[dataset_train.targets == t]) for t in range(classes)]
criterion = LDAMLoss(cls_num_list)
# 定义数据加载器
train_loader = DataLoader(dataset_train, batch_size=BATCH_SIZE, shuffle=True, num_workers=4, pin_memory=True)
test_loader = DataLoader(dataset_test, batch_size=BATCH_SIZE, shuffle=False, num_workers=4, pin_memory=True)
# 训练模型
best_acc = 0.0
for epoch in range(start_epoch, EPOCHS + 1):
model.train()
train_loss = 0.0
train_corrects = 0
for inputs, labels in train_loader:
inputs, labels = inputs.to(DEVICE), labels.to(DEVICE)
if use_dp:
inputs, labels = dp(inputs, labels)
if use_amp:
with amp.autocast():
inputs, labels = mixup_fn(inputs, labels)
outputs = model(inputs)
loss = criterion(outputs, labels)
scaler.scale(loss).backward()
scaler.unscale_(optimizer)
torch.nn.utils.clip_grad_norm_(model.parameters(), CLIP_GRAD)
scaler.step(optimizer)
scaler.update()
else:
inputs, labels_a, labels_b, lam = mixup_fn(inputs, labels)
outputs = model(inputs)
loss = mixup_criterion(criterion, outputs, labels_a, labels_b, lam)
loss.backward()
torch.nn.utils.clip_grad_norm_(model.parameters(), CLIP_GRAD)
optimizer.step()
optimizer.zero_grad()
train_loss += loss.item() * inputs.size(0)
_, preds = torch.max(outputs, 1)
train_corrects += torch.sum(preds == labels.data)
train_loss /= len(dataset_train)
train_acc = train_corrects.double() / len(dataset_train)
model.eval()
test_loss = 0.0
test_corrects = 0
with torch.no_grad():
for inputs, labels in test_loader:
inputs, labels = inputs.to(DEVICE), labels.to(DEVICE)
outputs = model(inputs)
loss = criterion(outputs, labels)
test_loss += loss.item() * inputs.size(0)
_, preds = torch.max(outputs, 1)
test_corrects += torch.sum(preds == labels.data)
test_loss /= len(dataset_test)
test_acc = test_corrects.double() / len(dataset_test)
# 更新最佳模型
if test_acc > best_acc:
if use_ema:
ema_model.load_state_dict(model.state_dict())
best_acc = test_acc
# 更新学习率
scheduler.step()
# 打印训练结果
print('Epoch [{}/{}], Train Loss: {:.4f}, Train Acc: {:.4f}, Test Loss: {:.4f}, Test Acc: {:.4f}'.format(
epoch, EPOCHS, train_loss, train_acc, test_loss, test_acc))
```
相关推荐
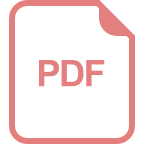













