用c++判断轮廓与直线是否相交
时间: 2023-05-18 14:06:07 浏览: 197
可以使用 OpenCV 库中的函数 cv::intersectConvexConvex() 来判断轮廓与直线是否相交。该函数可以判断两个凸多边形是否相交,因此需要将直线转换为一个长度为无穷大的矩形,然后将轮廓转换为一个凸多边形,最后调用该函数即可。具体实现可以参考以下代码:
```c
#include <opencv2/opencv.hpp>
using namespace cv;
bool isIntersect(const std::vector<Point>& contour, const Vec4i& line)
{
// Convert line to a rectangle with infinite length
Rect rect(0, 0, INT_MAX, INT_MAX);
lineToRect(line, rect);
// Convert contour to a convex polygon
std::vector<Point> convexContour;
convexHull(contour, convexContour);
// Check if the convex polygon and the rectangle intersect
std::vector<Point2f> intersection;
bool isIntersect = intersectConvexConvex(convexContour, rect, intersection);
return isIntersect;
}
void lineToRect(const Vec4i& line, Rect& rect)
{
int x1 = line[0], y1 = line[1], x2 = line[2], y2 = line[3];
if (x1 == x2) {
rect.x = x1;
rect.y = 0;
rect.width = 1;
rect.height = INT_MAX;
} else if (y1 == y2) {
rect.x = 0;
rect.y = y1;
rect.width = INT_MAX;
rect.height = 1;
} else {
float k = (float)(y2 - y1) / (float)(x2 - x1);
float b = y1 - k * x1;
if (k > 0) {
rect.x = 0;
rect.y = b;
rect.width = INT_MAX;
rect.height = k * INT_MAX;
} else {
rect.x = INT_MAX;
rect.y = k * INT_MAX + b;
rect.width = INT_MAX;
rect.height = -k * INT_MAX;
}
}
}
```
其中,lineToRect() 函数将直线转换为一个矩形,isIntersect() 函数判断轮廓与直线是否相交。
阅读全文
相关推荐









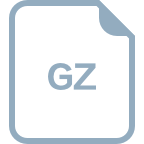