python爬虫提交表单
时间: 2023-11-08 09:49:46 浏览: 47
使用Python进行爬虫表单提交可以使用第三方库Requests。下面是一个简单的例子来说明如何使用Requests库进行表单提交:
```python
import requests
# 创建一个字典,包含表单数据
data = {'firstname': 'cck', 'lastname': 'Mi'}
# 发送POST请求并传递表单数据
response = requests.post("http://pythonscraping.com/files/processing.php", data=data)
# 打印响应内容
print(response.text)
```
上述代码中,我们首先导入了Requests库,然后创建了一个字典`data`,包含要提交的表单数据。接下来,我们使用`post`方法发送POST请求,并传递表单数据作为参数。最后,我们通过`response`对象的`text`属性获取到响应内容,并打印出来。
请注意,这只是一个基本的例子,实际情况中可能需要处理更复杂的表单数据和请求头信息。Requests库提供了许多其他功能,比如处理Cookie、设置请求头等,可以根据需要进行进一步的学习和使用。
相关问题
python爬虫火狐
你可以使用Selenium库来实现使用火狐浏览器进行Python爬虫。首先,你需要确保已经安装了Selenium库和火狐浏览器驱动器(geckodriver)。然后,可以按照以下步骤进行操作:
1. 导入所需的库:
```python
from selenium import webdriver
```
2. 创建一个Firefox浏览器对象:
```python
driver = webdriver.Firefox()
```
3. 使用浏览器对象打开网页:
```python
driver.get('http://example.com')
```
4. 执行一些操作,如查找元素、填写表单等:
```python
element = driver.find_element_by_id('some_id')
element.send_keys('some_text')
```
5. 关闭浏览器:
```python
driver.quit()
```
这样就可以使用火狐浏览器进行Python爬虫了。请注意,Selenium支持多种浏览器,包括火狐、Chrome、Safari等。
python爬虫图书
如果你对Python爬虫感兴趣,以下两本图书可能适合你:
1. 《Python网络爬虫权威指南第2版》是一本适合有编程背景的数据科学研究人员阅读的书籍。它提供了关于使用Python进行网络爬虫的全面指南,包括爬取网页、解析HTML和XML、处理表单、处理JavaScript等内容。这本书可以帮助你掌握Python爬虫的基本技术和方法,并将其应用于数据分析、操作和可视化等领域。
2. 《Python Cookbook(第3版)》是一本适合有一定编程基础的Python程序员阅读参考的书籍。虽然不是专门讲解爬虫的书籍,但其中的一些章节和方法可以帮助你编写高质量的Python代码,包括处理网络请求、解析HTML和XML、使用正则表达式等。这本书可以提供一些有关Python爬虫的实用技巧和建议,以帮助你更好地开发和调试爬虫程序。
希望这些推荐对你有帮助!<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [Python网络爬虫经典书籍推荐](https://blog.csdn.net/qq_37821082/article/details/118906752)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
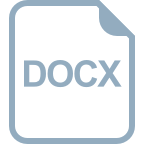
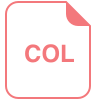
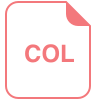
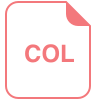
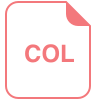
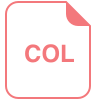







