#include <iostream> using namespace std; class Exception { public: virtual void showMessage()=0; }; class OutOfMemory : public Exception{ public: void showMessage() { cout << "Out of Memory!" << endl; } }; class RangeError : public Exception{ public: void showMessage() { cout << "Range Error!" << endl; } }; int main() { try { int n; cout<<"Input n(1<=n<=100):"; cin>>n; if(n<1||n>100) { if(n < 0){ throw OutOfMemory(); } else{ throw RangeError(); } } else{ cout<<"n:"<<n<<endl; } catch(Exception& e) { e.showMessage(); } }修正这个代码
时间: 2024-02-10 15:10:56 浏览: 29
以下是修正后的代码:
```cpp
#include <iostream>
using namespace std;
class Exception {
public:
virtual void showMessage() = 0;
};
class OutOfMemory : public Exception {
public:
void showMessage() {
cout << "Out of Memory!" << endl;
}
};
class RangeError : public Exception {
public:
void showMessage() {
cout << "Range Error!" << endl;
}
};
int main() {
try {
int n;
cout << "Input n(1<=n<=100):";
cin >> n;
if (n < 1 || n > 100) {
if (n < 0) {
throw OutOfMemory();
} else {
throw RangeError();
}
} else {
cout << "n:" << n << endl;
}
} catch (Exception& e) {
e.showMessage();
}
return 0;
}
```
修正后的代码增加了缺失的 `return 0;` 语句,并且将 `if (n < 1 || n > 100)` 的代码块中的 throw 语句放到了一个更内层的 if-else 结构中,以便判断 n 是否小于 0。此外,修正后的代码也加上了缺失的右括号。
相关推荐
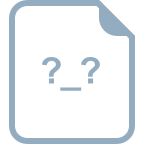















