编写一个函数,向某个地址进行POST请求,请求参数type为application/x-www-form-urlencoded
时间: 2024-06-09 09:07:30 浏览: 75
这里提供一个Python的示例代码,使用requests库向指定地址发送POST请求,传递参数类型为`application/x-www-form-urlencoded`:
```python
import requests
def post_request(url, data):
headers = {'Content-Type': 'application/x-www-form-urlencoded'}
response = requests.post(url, data=data, headers=headers)
return response.text
```
其中,`url`为请求地址,`data`为请求参数,类型为字典。例如,如果希望传递参数`user_id=123`和`user_name=John`,则`data`应该为`{'user_id': '123', 'user_name': 'John'}`。
调用示例:
```python
url = 'http://example.com/api'
data = {'user_id': '123', 'user_name': 'John'}
response = post_request(url, data)
print(response)
```
其中,`response`为请求的响应结果,类型为字符串。
相关问题
python fastapi 将请求头的Content-Type 从application/x-www-form-urlencoded改为application/json
在使用 FastAPI 中,你可以通过以下步骤将请求头的 Content-Type 从 "application/x-www-form-urlencoded" 更改为 "application/json":
1. 首先,确保你已经导入了 FastAPI 和 Request 相关的模块,例如:
```python
from fastapi import FastAPI, Request
```
2. 在你的路由处理函数中,将请求对象 `Request` 作为参数传入:
```python
@app.post("/your_endpoint")
async def your_route(request: Request):
# 在这里处理请求
```
3. 获取请求头中的 Content-Type,并将其更改为 "application/json":
```python
@app.post("/your_endpoint")
async def your_route(request: Request):
request.headers["content-type"] = "application/json"
# 在这里处理请求
```
通过这个步骤,你已经成功将请求头的 Content-Type 从 "application/x-www-form-urlencoded" 更改为 "application/json"。请确保在处理请求之前进行此更改,以便正确解析请求体中的 JSON 数据。
flutter 发送post请求 头为application/x-www-form-urlencoded json数据
在 Flutter 中发送 POST 请求并携带 `application/x-www-form-urlencoded` 格式的 JSON 数据,通常会涉及到 `http` 包的使用。首先确保已添加依赖:
```dart
dependencies:
http: ^0.13.3
```
然后可以创建一个函数来封装请求,这里是一个示例:
```dart
import 'package:http/http.dart' as http;
Future<http.Response> sendPostRequest(String url, dynamic jsonData) async {
// 将 JSON 转换为查询字符串格式
String encodedData = json.encode(jsonData);
Uri builtUri = Uri.parse(url + "?data=" + encodedData);
// 创建请求头,指定 content-type 为 application/x-www-form-urlencoded
final headers = <String, String>{
'Content-Type': 'application/x-www-form-urlencoded; charset=UTF-8',
};
// 发送 POST 请求
try {
return await http.post(
builtUri,
body: encodedData,
encoding: Encoding.getByName('UTF-8'),
headers: headers,
);
} catch (e) {
print('Error sending request: $e');
throw e;
}
}
```
在这个例子中,`jsonData` 是你要发送的 JSON 对象,你需要先将其转换为字符串格式。然后你可以像下面这样调用这个函数:
```dart
final data = {'key1': 'value1', 'key2': 'value2'};
final response = await sendPostRequest('https://your-api-url.com/endpoint', data);
// 检查响应状态
if (response.statusCode == 200) {
// 处理成功响应
} else {
// 处理错误响应
}
```
阅读全文
相关推荐
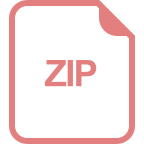
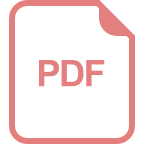













