已知E:\yxy\java路径下存在testin.txt文件。 (提示:需要先手动在E:\yxy\java路径下创建testin.txt文件,文件内要有适量字符内容) 编写程序实现,将testin.txt复制到D:\myfile文件夹中。D:\myfile不存在,需要创建,复制的文件名命名“copytestin.txt”.
时间: 2023-12-04 09:02:24 浏览: 32
好的,我可以帮你编写一个Java代码来实现这个功能。代码如下:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
String sourcePath = "E:\\yxy\\java\\testin.txt";
String destPath = "D:\\myfile\\copytestin.txt";
File sourceFile = new File(sourcePath);
File destFile = new File(destPath);
try {
if (!destFile.exists()) {
destFile.getParentFile().mkdirs();
destFile.createNewFile();
}
InputStream in = new FileInputStream(sourceFile);
OutputStream out = new FileOutputStream(destFile);
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
System.out.println("文件复制成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这段代码中,我们首先定义了源文件的路径和目标文件的路径。然后通过`File`类创建源文件和目标文件的实例。
在`try`块中,我们首先判断目标文件是否存在,如果不存在就创建该文件。然后定义`InputStream`和`OutputStream`来分别读取源文件和写入目标文件。接着定义一个缓冲区,用来存储读取到的字节。通过`read`方法从源文件中读取数据,并将数据写入目标文件中。最后关闭输入流和输出流,并提示复制成功。
你可以将这段代码保存为`FileCopy.java`文件,并在命令行中使用`javac`命令编译,然后使用`java`命令运行。当程序运行完成后,你就可以在`D:\myfile`文件夹中看到复制过来的`copytestin.txt`文件了。
相关推荐
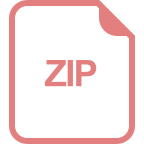
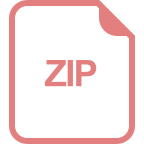
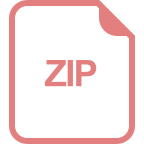








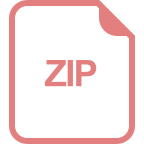
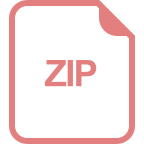
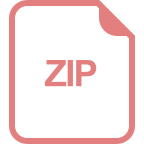
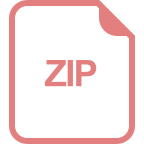
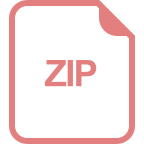
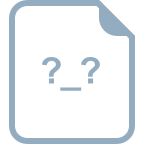