c语言统计相同单词的个数
时间: 2024-10-15 20:01:53 浏览: 46
在 C 语言中,统计相同单词的个数通常需要一些字符串处理步骤,包括读取输入、分割单词、存储并计数。这里提供一个简单的示例,假设我们没有使用库函数,而是手动处理:
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORDS 100 // 最大单词数量
#define MAX_WORD_LENGTH 50 // 单词最大长度
// 定义结构体来存储单词及其出现次数
typedef struct {
char word[MAX_WORD_LENGTH + 1];
int count;
} WordCount;
void split_and_count_words(char* input) {
char word[MAX_WORD_LENGTH + 1]; // 当前处理的单词
int words_processed = 0; // 已经处理的单词数量
int i;
// 分割单词并统计
for (i = 0; i <= strlen(input); i++) {
if (input[i] == ' ' || input[i] == '\n') { // 遇到空格或换行符
if (strlen(word) > 0) { // 如果当前单词非空
strcpy(WordCount[words_processed].word, word);
WordCount[words_processed].count = 1; // 初始计数为1
words_processed++;
}
word[0] = '\0'; // 清空当前单词
} else {
word[strlen(word)] = input[i]; // 继续添加字符
}
}
// 处理最后一个单词,如果还有剩余
if (strlen(word) > 0) {
strcpy(WordCount[words_processed].word, word);
WordCount[words_processed].count = 1; // 计数加一
words_processed++;
}
// 初始化剩余位置为0
for (; words_processed < MAX_WORDS; words_processed++) {
strcpy(WordCount[words_processed].word, "");
WordCount[words_processed].count = 0;
}
// 现在 WordCount 数组包含了所有单词及其出现次数
}
int main() {
char input[1024];
printf("请输入一段文本: ");
fgets(input, sizeof(input), stdin);
split_and_count_words(input);
int total_words = 0;
for (int i = 0; i < MAX_WORDS; i++) {
if (WordCount[i].count > 0) {
printf("%s出现了 %d 次\n", WordCount[i].word, WordCount[i].count);
total_words += WordCount[i].count;
}
}
printf("总共有 %d 个不同的单词.\n", total_words);
return 0;
}
```
这个程序会接收用户输入的文本,然后统计其中的不同单词及它们出现的次数。注意这只是一个基础版本,实际应用可能会需要更复杂的字符串处理和错误处理。
阅读全文
相关推荐
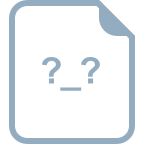
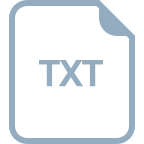
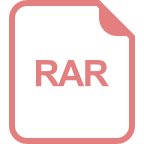

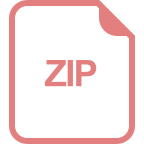
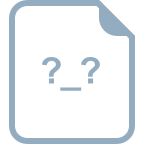
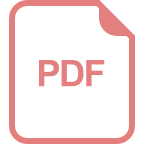
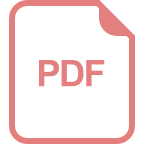
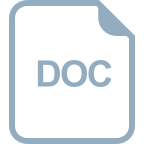
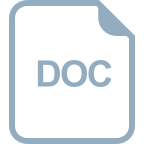
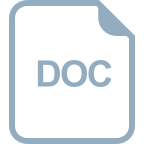
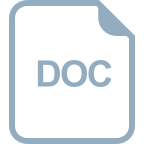
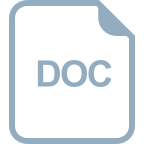



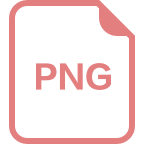