定义三个数组 char s1[20]; char s2[20]; char s3[20];
时间: 2023-11-15 13:05:07 浏览: 79
这三个数组都是字符数组,每个数组都有20个元素,可以存储20个字符。可以用以下方式定义:
```c
char s1[20];
char s2[20];
char s3[20];
```
需要注意的是,这三个数组没有被初始化,因此它们的初始值是未知的。如果需要初始化,可以使用以下方式:
```c
char s1[20] = "Hello";
char s2[20] = {'W', 'o', 'r', 'l', 'd', '\0'};
char s3[] = "Welcome to CSDN!";
```
第一种方式使用了字符串字面量来初始化,第二种方式使用了字符数组来初始化,第三种方式省略了数组长度,编译器会根据字符串字面量的长度来确定数组长度。
相关问题
note: expected ‘const char *’ but argument is of type ‘char’ char *mystrstr(const char* s1,const char* s2)
这个错误是因为在`main`函数中,您将`char`类型的字符串传递给了`mystrstr`函数,而`mystrstr`函数期望接收的是`const char*`类型的字符串。
为了解决这个问题,您需要将`s2`和`s3`声明为`const char*`类型的指针,而不是`char`类型的数组。
请将以下代码:
```c
char s2[] = "helloworld";
char s3[] = "ow";
```
改为:
```c
const char* s2 = "helloworld";
const char* s3 = "ow";
```
这样就能够解决这个问题。修正后的代码如下:
```c
#include<stdio.h>
#include<string.h>
char *mystrstr(const char* s1, const char* s2)
{
int i = 0, a = 0, c = 0;
int d = strlen(s2);
while (s1[i] != '\0' && a <= d - 1)
{
c = i;
while (s1[i] == s2[a])
{
i++;
a++;
}
if (s2[a] == '\0')
{
return (char*)(s1 + c);
}
i = c;
i++;
}
return NULL;
}
int main()
{
const char* s2 = "helloworld";
const char* s3 = "ow";
printf("%s\n", mystrstr(s2, s3));
return 0;
}
```
现在代码应该能够正确编译和运行了。
若三个字符串s1s2和s3其中s1=abcdef,s2=123456要求用字符数组现实将s1的内容复制到s3,即将s2的内容添加到s3的后面最后输出s3
好的,您可以使用strcpy()函数将s1的内容复制到s3,再使用strcat()函数将s2的内容添加到s3的后面。最后可以使用printf()函数输出s3的内容。下面是示例代码:
```
#include <stdio.h>
#include <string.h>
int main() {
char s1[] = "abcdef";
char s2[] = "123456";
char s3[13];
// 将s1的内容复制到s3
strcpy(s3, s1);
// 将s2的内容添加到s3的后面
strcat(s3, s2);
// 输出s3的内容
printf("%s\n", s3);
return 0;
}
```
输出结果为:
```
abcdef123456
```
相关推荐
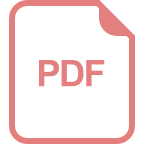
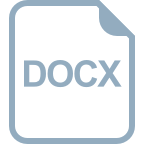
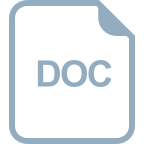












